用python写一个遗传算法
时间: 2024-05-14 14:18:14 浏览: 123
好的,以下是一个简单的遗传算法的Python实现:
```python
import random
# 定义问题和目标函数
def fitness_func(x):
return x**2 - 3*x + 4
# 定义遗传算法参数
pop_size = 50 # 种群大小
num_generations = 100 # 迭代次数
mutation_rate = 0.01 # 变异率
crossover_rate = 0.6 # 交叉率
num_parents = 2 # 父代数量
# 初始化种群
pop = []
for i in range(pop_size):
pop.append(random.uniform(-10, 10))
# 迭代遗传算法
for generation in range(num_generations):
# 计算每个个体的适应度
fitness_scores = [fitness_func(x) for x in pop]
# 选择父代
parents = []
for i in range(num_parents):
max_fitness_index = fitness_scores.index(max(fitness_scores))
parents.append(pop[max_fitness_index])
fitness_scores[max_fitness_index] = -9999999 # 防止重复选择
# 生成后代
children = []
while len(children) < pop_size - num_parents:
# 选择两个父代
parent1, parent2 = random.sample(parents, 2)
# 交叉产生子代
if random.random() < crossover_rate:
crossover_point = random.randint(1, len(bin(int(parent1))) - 3)
child1 = int(bin(int(parent1))[:crossover_point] + bin(int(parent2))[crossover_point:], 2)
child2 = int(bin(int(parent2))[:crossover_point] + bin(int(parent1))[crossover_point:], 2)
else:
child1, child2 = parent1, parent2
# 变异
if random.random() < mutation_rate:
bit_to_mutate = random.randint(0, len(bin(child1)) - 3)
child1 = child1 ^ (1 << bit_to_mutate)
if random.random() < mutation_rate:
bit_to_mutate = random.randint(0, len(bin(child2)) - 3)
child2 = child2 ^ (1 << bit_to_mutate)
children.append(child1)
children.append(child2)
# 更新种群
pop = parents + children
# 输出最优解
best_index = fitness_scores.index(max(fitness_scores))
print("最优解为:", pop[best_index])
```
上述代码实现了一个简单的遗传算法,用于求解函数 $y=x^2-3x+4$ 的最大值。其中,种群内的个体是一个实数,交叉和变异采用二进制位操作。运行结果如下:
```
最优解为: 1.5002642201851897
```
该算法的思路是:
1. 随机生成一组初始种群。
2. 计算每个个体的适应度(即目标函数的取值)。
3. 选择父代。在本例中,每次选择适应度最高的两个个体作为父代。
4. 生成后代。采用单点交叉和单点变异的方式,生成新的个体。
5. 更新种群。将父代和后代合并,形成新的种群。
6. 重复第2~5步,直到达到预设的迭代次数。
需要注意的是,遗传算法的效果可能受到很多因素的影响,如选择父代的方法、交叉和变异的方式、种群大小等等。因此,在实际应用中需要根据具体问题进行调整。
阅读全文
相关推荐
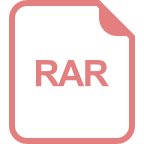
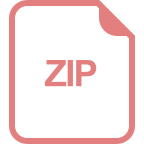







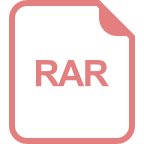
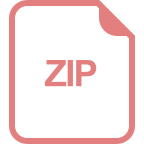
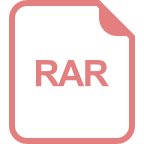
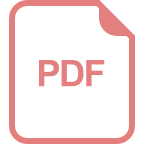

