给出一个简单班级考勤管理系统C语言代码,和部署过程
时间: 2024-03-27 21:41:29 浏览: 17
以下是一个简单班级考勤管理系统的C语言代码示例,包括添加学生信息、添加考勤记录、查询学生信息和考勤记录等功能。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENTS 50
struct student {
char name[30];
int id;
};
struct attendance {
int id;
char date[20];
char status[10];
};
struct student students[MAX_STUDENTS];
struct attendance attendances[MAX_STUDENTS];
int num_students = 0;
int num_attendances = 0;
void add_student() {
if (num_students >= MAX_STUDENTS) {
printf("The maximum number of students has been reached!\n");
return;
}
printf("Enter the name of the student: ");
scanf("%s", students[num_students].name);
printf("Enter the ID of the student: ");
scanf("%d", &students[num_students].id);
num_students++;
}
void add_attendance() {
if (num_attendances >= MAX_STUDENTS) {
printf("The maximum number of attendances has been reached!\n");
return;
}
int id;
char date[20], status[10];
printf("Enter the ID of the student: ");
scanf("%d", &id);
printf("Enter the date of the attendance (yyyy-mm-dd): ");
scanf("%s", date);
printf("Enter the status of the attendance: ");
scanf("%s", status);
int found = 0;
for (int i = 0; i < num_students; i++) {
if (students[i].id == id) {
found = 1;
break;
}
}
if (!found) {
printf("No student found with ID %d!\n", id);
return;
}
attendances[num_attendances].id = id;
strcpy(attendances[num_attendances].date, date);
strcpy(attendances[num_attendances].status, status);
num_attendances++;
}
void print_student(int i) {
printf("Name: %s, ID: %d\n", students[i].name, students[i].id);
}
void print_attendance(int i) {
printf("ID: %d, Date: %s, Status: %s\n", attendances[i].id, attendances[i].date, attendances[i].status);
}
void list_students() {
if (num_students == 0) {
printf("No students found!\n");
return;
}
printf("List of students:\n");
for (int i = 0; i < num_students; i++) {
print_student(i);
}
}
void list_attendances() {
if (num_attendances == 0) {
printf("No attendances found!\n");
return;
}
printf("List of attendances:\n");
for (int i = 0; i < num_attendances; i++) {
print_attendance(i);
}
}
int main() {
int choice;
while (1) {
printf("1. Add student\n");
printf("2. Add attendance\n");
printf("3. List students\n");
printf("4. List attendances\n");
printf("5. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch(choice) {
case 1:
add_student();
break;
case 2:
add_attendance();
break;
case 3:
list_students();
break;
case 4:
list_attendances();
break;
case 5:
exit(0);
default:
printf("Invalid choice!\n");
}
}
return 0;
}
```
部署过程:
1. 打开命令行终端,进入代码所在的文件夹。
2. 使用以下命令编译代码:`gcc -o attendance attendance.c`。
3. 运行程序:`./attendance`。
4. 根据程序提示,选择相应的功能进行操作。
注意:本代码仅作为示例,实际使用中可能需要根据具体需求进行修改和完善。
相关推荐
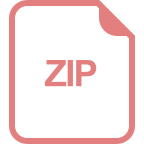
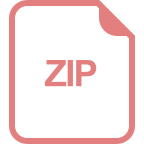
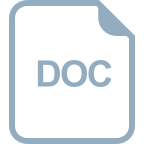














