lstm模型python代码
时间: 2023-09-03 17:08:19 浏览: 105
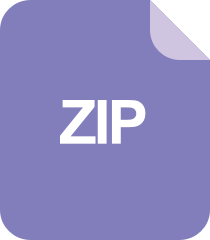
lstm_pythonLSTM_LSTM_源码.zip

以下是一个简单的LSTM模型的Python代码示例:
```
import tensorflow as tf
# 定义超参数
input_size = 28
timestep_size = 28
hidden_size = 128
layer_num = 2
class_num = 10
learning_rate = 0.001
batch_size = 128
# 定义输入和输出
X = tf.placeholder(tf.float32, [None, timestep_size, input_size])
Y = tf.placeholder(tf.float32, [None, class_num])
# 定义LSTM模型
lstm_cell = tf.nn.rnn_cell.BasicLSTMCell(hidden_size, forget_bias=1.0, state_is_tuple=True)
mlstm_cell = tf.nn.rnn_cell.MultiRNNCell([lstm_cell] * layer_num, state_is_tuple=True)
init_state = mlstm_cell.zero_state(batch_size, dtype=tf.float32)
outputs, state = tf.nn.dynamic_rnn(mlstm_cell, X, initial_state=init_state, time_major=False)
# 定义输出层
W = tf.Variable(tf.truncated_normal([hidden_size, class_num], stddev=0.1), dtype=tf.float32)
b = tf.Variable(tf.constant(0.1, shape=[class_num]), dtype=tf.float32)
output = tf.matmul(outputs[:, -1, :], W) + b
# 定义损失函数和优化器
cross_entropy = tf.nn.softmax_cross_entropy_with_logits_v2(logits=output, labels=Y)
loss = tf.reduce_mean(cross_entropy)
train_op = tf.train.AdamOptimizer(learning_rate).minimize(loss)
# 定义准确率
correct_prediction = tf.equal(tf.argmax(output, 1), tf.argmax(Y, 1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
# 开始训练
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for i in range(1000):
batch_xs, batch_ys = mnist.train.next_batch(batch_size)
batch_xs = batch_xs.reshape([batch_size, timestep_size, input_size])
sess.run(train_op, feed_dict={X: batch_xs, Y: batch_ys})
if i % 100 == 0:
acc = sess.run(accuracy, feed_dict={X: batch_xs, Y: batch_ys})
print('step %d, training accuracy %g' % (i, acc))
```
阅读全文
相关推荐
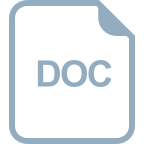
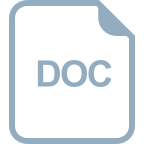















