pytorch 获取网络中间层输出
时间: 2023-01-31 11:01:50 浏览: 87
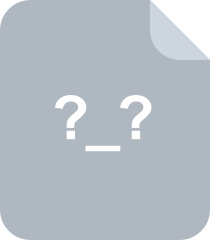
获取pytorch网络任意中间层输出
可以在网络的前向传播过程中获取中间层的输出。
首先,你需要获取网络中间层的对象,这可以通过网络的子模块获得。例如,如果你想获取网络中第二层的输出,你可以这样做:
```
layer2 = net.layer2
```
然后,在前向传播时,你可以调用这个层的 `forward` 方法,将输入传入它,并获取输出:
```
out = layer2(inputs)
```
示例代码:
```
import torch
import torch.nn as nn
# 定义一个简单的网络
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Conv2d(1, 6, 3)
self.conv2 = nn.Conv2d(6, 16, 3)
self.fc1 = nn.Linear(16 * 6 * 6, 120)
self.fc2 = nn.Linear(120, 84)
self.fc3 = nn.Linear(84, 10)
def forward(self, x):
x = self.conv1(x)
x = self.conv2(x)
x = x.view(-1, 16 * 6 * 6)
x = self.fc1(x)
x = self.fc2(x)
x = self.fc3(x)
return x
# 创建网络对象
net = Net()
# 获取第二层
layer2 = net.conv2
# 准备输入
inputs = torch.randn(1, 1, 32, 32)
# 获取第二层的输出
out = layer2(inputs)
print(out.shape) # 输出应该是 (1, 16, 30, 30)
```
希望这能帮到你!
阅读全文
相关推荐
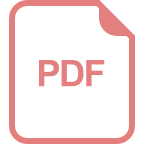
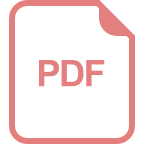














