用c#语言写一个贪吃蛇游戏
时间: 2023-07-20 12:24:55 浏览: 77
好的,我可以为您提供一个简单的 C# 贪吃蛇游戏的代码示例,您可以参考一下:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Threading;
namespace SnakeGame
{
class Program
{
static void Main(string[] args)
{
Console.CursorVisible = false;
Console.WindowHeight = 25;
Console.WindowWidth = 80;
Snake snake = new Snake();
Food food = new Food();
while (true)
{
if (Console.KeyAvailable)
{
ConsoleKeyInfo keyInfo = Console.ReadKey(true);
if (keyInfo.Key == ConsoleKey.LeftArrow && snake.direction != Direction.Right)
{
snake.direction = Direction.Left;
}
else if (keyInfo.Key == ConsoleKey.RightArrow && snake.direction != Direction.Left)
{
snake.direction = Direction.Right;
}
else if (keyInfo.Key == ConsoleKey.UpArrow && snake.direction != Direction.Down)
{
snake.direction = Direction.Up;
}
else if (keyInfo.Key == ConsoleKey.DownArrow && snake.direction != Direction.Up)
{
snake.direction = Direction.Down;
}
}
Console.Clear();
snake.Move();
snake.Draw();
if (snake.Collision(food))
{
snake.Eat(food);
food.Generate();
}
food.Draw();
if (snake.Collision())
{
Console.WriteLine("Game Over");
break;
}
Thread.Sleep(100);
}
}
}
public enum Direction
{
Left,
Right,
Up,
Down
}
public class Snake
{
public List<Point> body;
public Direction direction;
public Snake()
{
body = new List<Point>();
body.Add(new Point(40, 12));
body.Add(new Point(41, 12));
body.Add(new Point(42, 12));
direction = Direction.Left;
}
public void Move()
{
Point head = body.First();
Point newHead = new Point(head.x, head.y);
switch (direction)
{
case Direction.Left:
newHead.x--;
break;
case Direction.Right:
newHead.x++;
break;
case Direction.Up:
newHead.y--;
break;
case Direction.Down:
newHead.y++;
break;
}
body.Insert(0, newHead);
body.RemoveAt(body.Count - 1);
}
public bool Collision()
{
Point head = body.First();
if (head.x < 0 || head.x >= Console.WindowWidth || head.y < 0 || head.y >= Console.WindowHeight)
{
return true;
}
for (int i = 1; i < body.Count; i++)
{
if (head.x == body[i].x && head.y == body[i].y)
{
return true;
}
}
return false;
}
public bool Collision(Food food)
{
Point head = body.First();
if (head.x == food.location.x && head.y == food.location.y)
{
return true;
}
return false;
}
public void Eat(Food food)
{
Point head = body.First();
body.Insert(0, new Point(food.location.x, food.location.y));
}
public void Draw()
{
foreach (Point p in body)
{
Console.SetCursorPosition(p.x, p.y);
Console.Write("*");
}
}
}
public class Food
{
public Point location;
public Food()
{
Generate();
}
public void Generate()
{
Random random = new Random();
int x = random.Next(Console.WindowWidth);
int y = random.Next(Console.WindowHeight);
location = new Point(x, y);
}
public void Draw()
{
Console.SetCursorPosition(location.x, location.y);
Console.Write("@");
}
}
public class Point
{
public int x;
public int y;
public Point(int x, int y)
{
this.x = x;
this.y = y;
}
}
}
```
这个程序使用了 `Point` 类表示游戏场景中的点,`Snake` 类表示贪吃蛇,`Food` 类表示食物。在 `Main` 方法中,我们首先调整了控制台窗口的大小和光标的可见性,然后创建了一个 `Snake` 和一个 `Food` 对象。然后进入了游戏循环,不断更新蛇和食物的位置,并检查碰撞和键盘输入等事件。如果蛇和食物发生了碰撞,就让蛇吃下食物,并随机生成新的食物。如果蛇和边界或自身发生了碰撞,游戏就结束了。
相关推荐
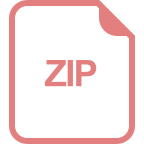
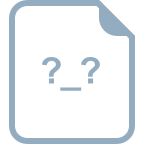











