用python的mayavi写一个窗口连续显示的代码
时间: 2023-04-10 18:00:57 浏览: 81
可以的,以下是一个简单的示例代码:
```python
import numpy as np
from mayavi import mlab
# 创建数据
x, y, z = np.mgrid[-5:5:100j, -5:5:100j, -5:5:100j]
s = np.sin(x*y*z)/(x*y*z)
# 创建场景
fig = mlab.figure()
# 创建初始图像
src = mlab.pipeline.scalar_field(s)
vol = mlab.pipeline.volume(src)
# 连续更新图像
for i in range(100):
# 更新数据
s = np.sin(x*y*z + i*np.pi/50)/(x*y*z)
src.mlab_source.scalars = s
# 更新图像
vol.update_pipeline()
# 等待一段时间
mlab.pause(0.1)
# 显示场景
mlab.show()
```
这个代码使用了 Mayavi 库创建了一个三维数据的可视化场景,并且使用了一个循环来连续更新数据和图像。你可以根据自己的需求修改这个代码。
相关问题
用python的mayavi写一个窗口连续显示3d点的代码
可以回答这个问题。以下是一个可能的代码示例:
```python
import numpy as np
from mayavi import mlab
# 生成一些随机的3D点
x, y, z = np.random.rand(3, 10)
# 创建一个3D场景
fig = mlab.figure()
# 绘制初始的点云
points = mlab.points3d(x, y, z, color=(1, 0, 0), mode='sphere', scale_factor=0.1)
# 定义一个更新函数,每次调用会更新点云的位置
def update_points():
# 生成新的随机点
x, y, z = np.random.rand(3, 10)
# 更新点云的位置
points.mlab_source.set(x=x, y=y, z=z)
# 创建一个定时器,每隔0.1秒调用一次更新函数
timer = mlab.timer_callback(update_points, interval=100)
# 显示场景
mlab.show()
```
这个代码会生成一个3D场景,并在场景中显示一些随机的点。然后,它会创建一个定时器,每隔0.1秒调用一次更新函数,更新点云的位置。这样,窗口就会连续显示3D点。
用python和mayavi写一个展示氢原子轨道的程序
我可以为你提供一个可以使用python和mayavi来展示氢原子轨道的程序的建议:首先,使用python的scipy和numpy模块来构建数值模拟氢原子的轨道。其次,使用mayavi来画出氢原子轨道的3D可视化图像。最后,使用python的matplotlib模块来绘制氢原子轨道的投影图像。
相关推荐
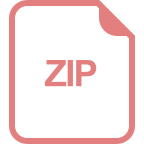
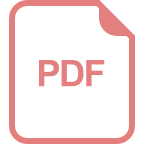
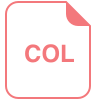
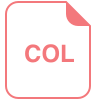
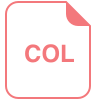
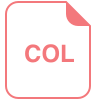
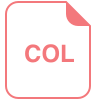







