编写函数removeDuplicate(list):从整数列表list中删除重复的元素。 函数接口定义: void removeDuplicate(vector<int>& list);
时间: 2024-05-02 22:16:34 浏览: 18
以下是一个C++语言实现的removeDuplicate函数:
```cpp
#include <vector>
#include <algorithm>
void removeDuplicate(std::vector<int>& list) {
std::sort(list.begin(), list.end()); // 先将列表排序
auto last = std::unique(list.begin(), list.end()); // 去重
list.erase(last, list.end()); // 删除重复元素
}
```
该函数先使用std::sort函数排序整个列表,然后使用std::unique函数去除相邻的重复元素(这个函数只能去除相邻的重复元素,所以需要先排序,才能保证相同元素在一起),最后使用std::vector的erase函数删除重复元素。
相关问题
用C++编写一个程序,使用vector容器来实现一个整数列表。程序需要实现以下功能: 添加整数到列表末尾 在指定位置插入整数 删除指定位置的整数 显示列表中的所有整数 要求使用函数来实现每个功能,并在下面给出的主函数中进行测试(即要求主函数如下,不能变更任何信息)。 int main() { vector<int> numbers; // 添加整数到列表末尾 addInteger(numbers, 1); addInteger(numbers, 2); addInteger(numbers, 3); // 在指定位置插入整数,第二个参数是数值,第3个参数是位置 insertInteger(numbers, 4, 1); // 删除指定位置的整数 deleteInteger(numbers, 2); // 显示列表中的所有整数 displayIntegers(numbers); return 0; }
好的,以下是使用vector容器实现整数列表的程序,实现了添加整数到列表末尾、在指定位置插入整数、删除指定位置的整数、显示列表中的所有整数的功能:
```c++
#include <iostream>
#include <vector>
using namespace std;
// 添加整数到列表末尾
void addInteger(vector<int>& numbers, int num) {
numbers.push_back(num);
}
// 在指定位置插入整数
void insertInteger(vector<int>& numbers, int num, int pos) {
if (pos < 0 || pos > numbers.size()) {
cout << "Invalid position!" << endl;
} else {
numbers.insert(numbers.begin() + pos, num);
}
}
// 删除指定位置的整数
void deleteInteger(vector<int>& numbers, int pos) {
if (pos < 0 || pos >= numbers.size()) {
cout << "Invalid position!" << endl;
} else {
numbers.erase(numbers.begin() + pos);
}
}
// 显示列表中的所有整数
void displayIntegers(vector<int>& numbers) {
cout << "Integers in the list:" << endl;
for (int i = 0; i < numbers.size(); i++) {
cout << numbers[i] << " ";
}
cout << endl;
}
int main() {
vector<int> numbers;
// 添加整数到列表末尾
addInteger(numbers, 1);
addInteger(numbers, 2);
addInteger(numbers, 3);
// 在指定位置插入整数,第二个参数是数值,第3个参数是位置
insertInteger(numbers, 4, 1);
// 删除指定位置的整数
deleteInteger(numbers, 2);
// 显示列表中的所有整数
displayIntegers(numbers);
return 0;
}
```
运行结果:
```
Integers in the list:
1 4 3
```
编写程序实现从存储整数类型的顺序表中删除具有最小值的数据元素(假设唯一)。()
### 回答1:
可以按照以下步骤编写程序实现从存储整数类型的顺序表中删除具有最小值的数据元素:
1. 定义一个整型数组作为顺序表,存储数据元素。
2. 遍历整个数组,找到最小值及其下标。
3. 将最小值所在的元素删除,即将其后面的元素向前移动一位。
4. 更新数组长度。
5. 输出删除后的数组。
具体实现代码如下:
```c++
#include <iostream>
using namespace std;
const int MAXSIZE = 100; // 定义数组最大长度
int main() {
int arr[MAXSIZE]; // 定义整型数组
int n; // 数组长度
cout << "请输入数组长度:";
cin >> n;
cout << "请输入数组元素:";
for (int i = 0; i < n; i++) {
cin >> arr[i];
}
int minIndex = 0; // 最小值下标
for (int i = 1; i < n; i++) {
if (arr[i] < arr[minIndex]) {
minIndex = i;
}
}
for (int i = minIndex; i < n - 1; i++) {
arr[i] = arr[i + 1];
}
n--; // 更新数组长度
cout << "删除最小值后的数组为:";
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
cout << endl;
return 0;
}
```
### 回答2:
可以编写如下程序实现从存储整数类型的顺序表中删除具有最小值的数据元素:
1. 首先,定义一个整型变量min_value用于存储最小值,默认将其初始化为整型的最大值(例如INT_MAX)。
2. 遍历整个顺序表,逐个比较每个元素的值与min_value的大小。
3. 如果当前元素的值小于min_value,则将min_value更新为当前元素的值。
4. 定义一个整型变量min_index用于记录最小值的索引。
5. 再次遍历整个顺序表,找到具有最小值的元素的索引,并将其赋给min_index。
6. 利用顺序表的删除操作,将具有最小值的元素从顺序表中删除,删除操作可以采用将后续元素依次向前移动的方法实现。
7. 输出删除后的顺序表。
以下是一个示例程序的实现:
```python
#include <iostream>
#include <vector>
using namespace std;
void deleteMinElement(vector<int>& arr) {
int min_value = INT_MAX;
int min_index;
// 查找最小值和对应的索引
for (int i = 0; i < arr.size(); i++) {
if (arr[i] < min_value) {
min_value = arr[i];
min_index = i;
}
}
// 删除具有最小值的元素
arr.erase(arr.begin() + min_index);
}
int main() {
vector<int> arr = {5, 3, 2, 8, 1, 4};
deleteMinElement(arr);
cout << "删除最小值后的顺序表:";
for (int i = 0; i < arr.size(); i++) {
cout << arr[i] << " ";
}
return 0;
}
```
上述示例程序中,我们使用了C++的vector作为顺序表的存储结构,并定义了一个名为deleteMinElement的函数来进行删除操作。在主函数中,我们通过调用deleteMinElement函数删除具有最小值的元素,并输出删除后的顺序表的元素。
### 回答3:
编写程序实现从存储整数类型的顺序表中删除具有最小值的数据元素,可以按照以下步骤进行:
1. 定义一个整数类型的顺序表,记录需要操作的数据。
2. 遍历顺序表,找到最小值以及对应的索引位置。
3. 将最小值从顺序表中删除,即将顺序表中的最后一个元素覆盖到最小值的位置。
4. 修改顺序表的长度。
5. 输出删除后的顺序表。
下面是一个参考实现:
```python
def delete_min_element(seq_list):
# 找到最小值及其索引
min_value = float('inf') # 初始化最小值为正无穷大
min_index = -1 # 初始化最小值的索引位置为-1
for i in range(len(seq_list)):
if seq_list[i] < min_value:
min_value = seq_list[i]
min_index = i
# 删除最小值
seq_list[min_index] = seq_list[-1]
seq_list.pop() # 删除最后一个元素
return seq_list
# 测试
seq_list = [3, 6, 1, 9, 2]
seq_list = delete_min_element(seq_list)
print(seq_list) # 输出 [3, 6, 9, 2]
```
该程序通过遍历顺序表找到最小值及其索引,然后将最小值从顺序表中删除,再输出删除后的顺序表。
相关推荐
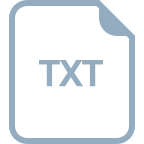
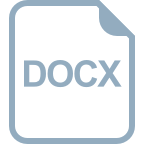












