def attention_sublayers(self, feats, embedding_layers, latent): feats = feats.view((feats.size(0), self.k, -1)) feats = feats.transpose(dim0=1, dim1=2) feats = feats + latent.unsqueeze(1) feats = feats.transpose(dim0=1, dim1=2) feats = embedding_layers(feats).squeeze(-1) p = F.softmax(feats, dim=1) return p def forward(self, x): conv2_2 = self.conv2_2(x) conv3_4 = self.conv3_4(conv2_2) conv4_4 = self.conv4_4(conv3_4) conv5_4 = self.conv5_4(conv4_4) x = F.relu(self.fc4(self.fc_layers(self.tail_layer(conv5_4).view(-1, 25088)))) attr = self.bn1(x[:, :self.k]) latent = self.bn2(x[:, self.k:]) feats_0 = self.extract_0(conv2_2) feats_1 = self.extract_1(conv3_4) feats_2 = self.extract_2(conv4_4) feats_3 = self.extract_3(conv5_4) # N x k x 14 x 14 p_0 = self.attention_sublayers(feats_0, self.fc0, latent) p_1 = self.attention_sublayers(feats_1, self.fc1, latent) p_2 = self.attention_sublayers(feats_2, self.fc2, latent) p_3 = self.attention_sublayers(feats_3, self.fc3, latent) # N x k p = p_0 + p_1 + p_2 + p_3
时间: 2023-07-14 20:14:04 浏览: 95
这段代码是一个神经网络模型的前向传播函数,该模型使用了注意力机制(attention mechanism)来提高其性能。
首先,输入 x 经过几个卷积层和全连接层得到一个特征向量 x,我们可以把这个特征向量分成两部分,一部分用于属性预测,一部分用于潜在特征的计算。
接下来,我们从不同层的卷积特征图中提取出一些特征,然后使用 attention_sublayers 函数来计算每个特征的权重。这里的 attention_sublayers 函数接受一个特征张量 feats,一个嵌入层列表 embedding_layers 和一个潜在特征向量 latent。它将特征张量 feats 调整为一个三维张量,然后加上潜在特征向量 latent,再把它调整回原来的形状。接着,它将调整后的特征张量 feats 传入嵌入层列表 embedding_layers 中,将最后一维压缩掉,得到一个二维张量。最后,它对这个二维张量的第二个维度应用 softmax 函数,得到每个特征的权重。
最后,将所有特征的权重相加,得到一个一维张量 p,它表示每个特征的重要性。
相关问题
class MemoryEncoding(nn.Module): def __init__(self, in_feats, out_feats, mem_size): super(MemoryEncoding, self).__init__() self.in_feats = in_feats self.out_feats = out_feats self.mem_size = mem_size self.linear_coef = nn.Linear(in_feats, mem_size, bias=True) self.act = nn.LeakyReLU(0.2, inplace=True) self.linear_w = nn.Linear(mem_size, out_feats * in_feats, bias=False)
这段代码定义了一个名为 `MemoryEncoding` 的类,它是一个继承自 `nn.Module` 的神经网络模块。
在 `__init__` 方法中,它接受三个参数:`in_feats`(输入特征的大小)、`out_feats`(输出特征的大小)和 `mem_size`(内存大小)。然后,它调用 `super()` 函数来初始化基类 `nn.Module`,并将输入参数保存为类的属性。
接下来,它创建了几个神经网络层:
- `self.linear_coef` 是一个线性层,将输入特征的大小 `in_feats` 转换为权重系数 `mem_size`。这个线性层具有偏置项。
- `self.act` 是一个带有负斜率的泄露线性整流单元(LeakyReLU)激活函数。它用于引入非线性性并提高模型的表达能力。
- `self.linear_w` 是另一个线性层,将权重系数 `mem_size` 转换为权重 `out_feats * in_feats`。这个线性层没有偏置项。
这些神经网络层将在模块的前向传播过程中使用,用于对输入数据进行编码和生成权重。
class MemoryEncoding(nn.Module): def __init__(self, in_feats, out_feats, mem_size): #调用 super() 函数来初始化基类 nn.Module,并将输入参数保存为类的属性。 super(MemoryEncoding, self).__init__() self.in_feats = in_feats self.out_feats = out_feats self.mem_size = mem_size self.linear_coef = nn.Linear(in_feats, mem_size, bias=True) self.act = nn.LeakyReLU(0.2, inplace=True) self.linear_w = nn.Linear(mem_size, out_feats * in_feats, bias=False) def get_weight(self, x): coef = self.linear_coef(x) if self.act is not None: coef = self.act(coef) w = self.linear_w(coef) w = w.view(-1, self.out_feats, self.in_feats) return w def forward(self, h_dst, h_src): w = self.get_weight(h_dst) res = torch.einsum('boi, bi -> bo', w, h_src) return res 举个例子
好的,我将给出一个具体的示例,演示如何使用 `MemoryEncoding` 类进行前向传播计算。
```python
import torch
import torch.nn as nn
# 定义输入张量的大小和内存大小
in_feats = 10
out_feats = 5
mem_size = 3
# 创建 MemoryEncoding 实例
memory_encoding = MemoryEncoding(in_feats, out_feats, mem_size)
# 创建示例输入张量
batch_size = 2
dst_feats = in_feats
src_feats = in_feats
h_dst = torch.randn(batch_size, dst_feats)
h_src = torch.randn(batch_size, src_feats)
# 执行前向传播
output = memory_encoding.forward(h_dst, h_src)
print(output.shape) # 输出形状为 (batch_size, out_feats)
```
在这个示例中,我们首先导入了 PyTorch 的 `nn` 模块,并定义了输入张量的大小和内存大小。然后,我们创建了一个 `MemoryEncoding` 类的实例 `memory_encoding`。
接下来,我们使用随机生成的张量 `h_dst` 和 `h_src` 执行前向传播计算,并将结果保存在 `output` 变量中。最后,我们打印出输出张量的形状。
请注意,这只是一个示例,实际使用时需要根据具体问题和数据调整输入张量的大小和值。
阅读全文
相关推荐
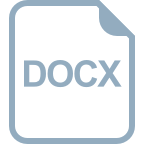
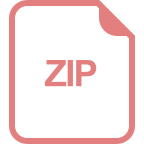
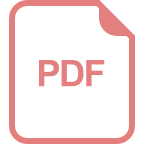













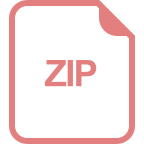