用C++编程三角函数计算器
时间: 2023-12-11 13:04:43 浏览: 179
下面是一个简单的C++程序,用于计算三角函数:
```
#include <iostream>
#include <cmath>
using namespace std;
int main()
{
double angle;
cout << "请输入角度:";
cin >> angle;
double radians = angle * M_PI / 180.0;
cout << "sin(" << angle << ") = " << sin(radians) << endl;
cout << "cos(" << angle << ") = " << cos(radians) << endl;
cout << "tan(" << angle << ") = " << tan(radians) << endl;
return 0;
}
```
该程序使用了 `<cmath>` 库中的 `sin`、`cos` 和 `tan` 函数,这些函数可以计算给定角度的正弦、余弦和正切值。程序先提示用户输入角度,然后将角度转换为弧度,并计算三角函数的值。运行程序后,用户可以输入任意角度,并得到对应的三角函数值。
相关问题
用C++实现一个计算器程序,该计算器支持以下功能: (1)支持加、减、乘、除四则运算 (2)支持带括号的优先级运算 (3)支持常用数学函数表达式 (开方、幂、三角函数、对数等) (4)自己给计算器添加
要实现一个C++计算器程序,我们可以采用递归解析器或者简单的语法分析器,利用栈数据结构处理运算顺序和括号。这里提供一个基础框架的示例,不包括所有复杂的数学函数,因为这涉及到库函数的引入和更深入的编程技术。
```cpp
#include <iostream>
#include <stack>
#include <string>
#include <cmath>
// 定义运算符优先级
enum class Priority {
LEFT_PAREN,
NUMBER,
ADD,
SUBTRACT,
MULTIPLY,
DIVIDE,
FUNCTION // 对于自定义函数的优先级
};
// 自己定义的数学函数处理
double custom_function(const std::string& func, double num) {
if (func == "sqrt") return sqrt(num);
else if (func == "pow") return pow(num, 2); // 示例仅包含平方
// 更多函数需要额外的实现
}
class Calculator {
public:
double calculate(const std::string& expression) {
std::stack<double> values;
std::stack<int> operators;
for (char c : expression) {
if (isdigit(c)) { // 处理数字
int val = 0;
while (isdigit(c)) {
val = 10 * val + c - '0';
++c; // 遍历字符流
}
values.push(val);
} else if (c == '+' || c == '-' || c == '*' || c == '/') { // 处理运算符
while (!operators.empty() && hasHigherPriority(c, operators.top())) {
// 执行栈顶运算
perform_operation(values, operators);
}
operators.push(c);
} else if (c == '(') { // 开始新的运算子表达式
operators.push(Priority::LEFT_PAREN);
} else if (c == ')') { // 结束当前运算子表达式并计算
while (!operators.top() != Priority::LEFT_PAREN) {
perform_operation(values, operators);
}
operators.pop();
} else if (c == '.') { // 如果遇到小数点,则继续读取下一个数字
continue;
} else if (isalpha(c)) { // 检查是否是自定义函数
if (custom_functions.find(c) != custom_functions.end()) {
double arg = values.top(); // 获取第一个操作数
values.pop();
values.push(custom_function(c, arg));
}
}
}
// 处理剩余未计算的运算
while (!operators.empty()) {
perform_operation(values, operators);
}
return values.top(); // 返回最终结果
}
private:
bool hasHigherPriority(char op1, int priority2) const {
switch (op1) {
case '+':
case '-':
return priority2 <= Priority::MULTIPLY;
case '*':
case '/':
return priority2 <= Priority::DIVIDE;
default:
return false;
}
}
void perform_operation(std::stack<double>& values, std::stack<int>& operators) {
int operator_ = operators.top();
double operand2 = values.top();
values.pop();
double operand1 = values.top();
values.pop();
switch (operator_) {
case Priority::ADD:
values.push(operand1 + operand2);
break;
case Priority::SUBTRACT:
values.push(operand1 - operand2);
break;
case Priority::MULTIPLY:
values.push(operand1 * operand2);
break;
case Priority::DIVIDE:
values.push(operand1 / operand2);
break;
// 自定义函数部分...
}
operators.pop();
}
std::map<char, double (*)(double, double)> custom_functions = {
{'s', &custom_function}, // 示例:平方根
{'p', &custom_function} // 示例:平方
};
};
int main() {
Calculator calc;
std::string expr = "2 + 3 * (4 - 5) / 6 + sqrt(9)";
double result = calc.calculate(expr);
std::cout << "Result: " << result << std::endl;
return 0;
}
```
阅读全文
相关推荐
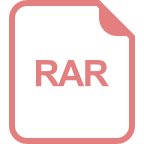
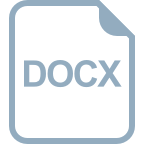
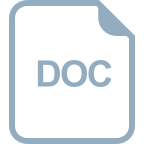
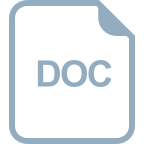
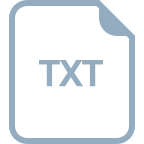
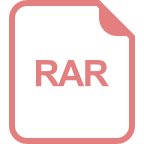
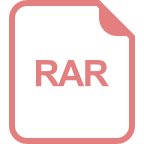
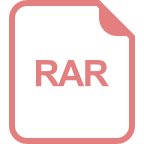
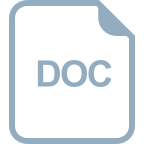
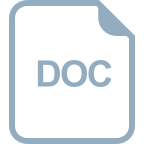
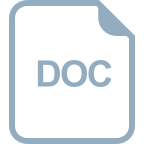
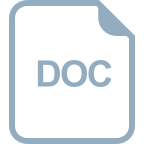
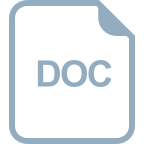
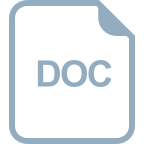