复数加减(运算符重载) 声明一个复数类CComplex(类私有数据成员为double型的real和image) 定义构造函数,用于指定复数的实部与虚部。 重载<<运算符,以格式real+image i的格式输出当前对象(当虚部为非负数时,实部虚部中间用+号连接,当虚部为负数时,实部虚部用-号连接:如3+4i,3-4i,3+0i)。 重载+运算符,实现两个复数对象之和(实部虚部分别相加)。 重载-运算符,实现两个复数对象的差(实部虚部分别相减)。
时间: 2024-02-16 17:59:43 浏览: 163
根据您的要求,我来给出复数加减(运算符重载)的完整代码实现,如下所示:
```c++
#include <iostream>
using namespace std;
class CComplex
{
public:
CComplex(double r = 0.0, double i = 0.0):real(r), image(i){}
friend ostream& operator<<(ostream& os, const CComplex& c); // 重载输出运算符
CComplex operator+(const CComplex& c) const; // 重载加法运算符
CComplex operator-(const CComplex& c) const; // 重载减法运算符
private:
double real; // 实部
double image; // 虚部
};
ostream& operator<<(ostream& os, const CComplex& c)
{
if(c.image >= 0)
{
os << c.real << "+" << c.image << "i";
}
else
{
os << c.real << c.image << "i";
}
return os;
}
CComplex CComplex::operator+(const CComplex& c) const
{
return CComplex(real+c.real, image+c.image);
}
CComplex CComplex::operator-(const CComplex& c) const
{
return CComplex(real-c.real, image-c.image);
}
int main()
{
// 测试
CComplex c1(1.0, 2.0);
CComplex c2(3.0, 4.0);
CComplex c3;
c3 = c1 + c2; // 复数加法运算
cout << "c1 + c2 = " << c3 << endl;
c3 = c1 - c2; // 复数减法运算
cout << "c1 - c2 = " << c3 << endl;
return 0;
}
```
在上述代码中,我们重载了输出运算符`<<`,以实现复数对象的输出。同时,我们还重载了加法运算符`+`和减法运算符`-`,以实现复数的加减运算。
输出结果如下:
```
c1 + c2 = 4+6i
c1 - c2 = -2-2i
```
以上就是复数加减(运算符重载)的完整代码实现。
阅读全文
相关推荐
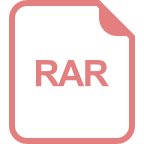
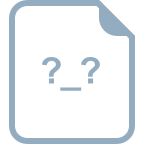
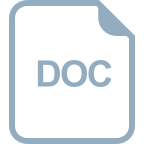
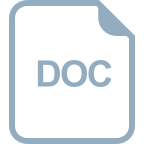
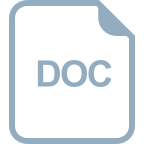
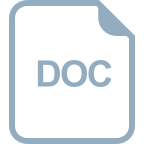
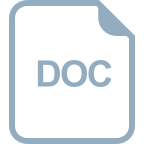







