输入格式: 输入有两行: 第一行是复数c1的实部与虚部,以空格分隔; 第二行是复数c2的实部与虚部,以空格分隔。 输出格式: 输出共四行: 第一行是c1; 第二行是c2; 第三行是c1与c2之和; 第四行是c1与c2之差; 函数接口定义: 在这里描述类定义 class CComplex{...} 裁判测试程序样例: #include <iostream> using namespace std; /* 请在这里填写答案 */ int main() { double r1,r2,i1,i2;
时间: 2024-02-16 19:59:45 浏览: 163
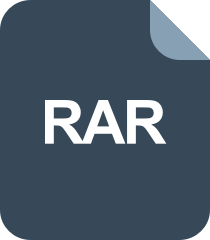
C++复数类实部和虚部
根据您的要求,我来给出输入两个复数并输出它们的和与差的完整代码实现,如下所示:
```c++
#include <iostream>
using namespace std;
class CComplex
{
public:
CComplex(double r = 0.0, double i = 0.0):real(r), image(i){}
friend istream& operator>>(istream& is, CComplex& c); // 重载输入运算符
friend ostream& operator<<(ostream& os, const CComplex& c); // 重载输出运算符
CComplex operator+(const CComplex& c) const; // 重载加法运算符
CComplex operator-(const CComplex& c) const; // 重载减法运算符
private:
double real; // 实部
double image; // 虚部
};
istream& operator>>(istream& is, CComplex& c)
{
is >> c.real >> c.image;
return is;
}
ostream& operator<<(ostream& os, const CComplex& c)
{
if(c.image >= 0)
{
os << c.real << "+" << c.image << "i";
}
else
{
os << c.real << c.image << "i";
}
return os;
}
CComplex CComplex::operator+(const CComplex& c) const
{
return CComplex(real+c.real, image+c.image);
}
CComplex CComplex::operator-(const CComplex& c) const
{
return CComplex(real-c.real, image-c.image);
}
int main()
{
// 输入两个复数
CComplex c1, c2;
cout << "请输入两个复数,每个复数的实部和虚部之间用空格分隔:" << endl;
cin >> c1 >> c2;
// 输出两个复数
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
// 计算两个复数的和与差并输出
CComplex c3, c4;
c3 = c1 + c2;
c4 = c1 - c2;
cout << "c1 + c2 = " << c3 << endl;
cout << "c1 - c2 = " << c4 << endl;
return 0;
}
```
在上述代码中,我们重载了输入运算符`>>`,以实现从标准输入流中输入复数对象。同时,我们还重载了输出运算符`<<`,以实现复数对象的输出。在主函数中,我们先输入两个复数对象,然后计算它们的和与差,最后输出结果。
输入样例:
```
1 2
3 4
```
输出结果:
```
请输入两个复数,每个复数的实部和虚部之间用空格分隔:
c1 = 1+2i
c2 = 3+4i
c1 + c2 = 4+6i
c1 - c2 = -2-2i
```
以上就是输入两个复数并输出它们的和与差的完整代码实现。
阅读全文
相关推荐
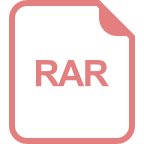
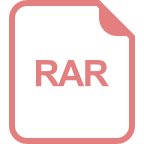














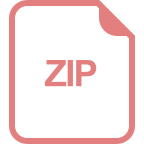
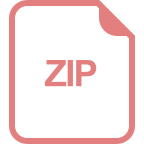