给定(某条曲线上)多个点,选择合适的函数(可以是一个也可以多个)构造它们的最小二乘拟合,比较拟合函数和原函数的图像及误差。C++
时间: 2023-11-28 12:48:30 浏览: 61
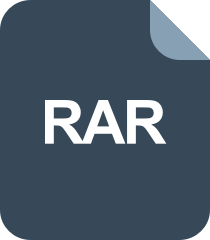
kuangjia.rar_visual c_拟合_曲面_曲面 最小二乘_曲面拟合C++
在 C++ 中,可以使用 Eigen 库来实现最小二乘拟合。以下是一个简单的示例代码,它使用多项式函数来拟合一些给定的点:
```c++
#include <iostream>
#include <Eigen/Dense>
using namespace Eigen;
int main()
{
// Define the points to be fitted
std::vector<double> x = {0.0, 1.0, 2.0, 3.0, 4.0, 5.0};
std::vector<double> y = {1.0, 2.0, 3.0, 4.0, 5.0, 6.0};
// Define the degree of the polynomial to be fitted
int degree = 2;
// Construct the Vandermonde matrix
MatrixXd A(x.size(), degree + 1);
for (int i = 0; i < x.size(); i++) {
for (int j = 0; j < degree + 1; j++) {
A(i, j) = pow(x[i], j);
}
}
// Construct the right-hand side vector
VectorXd b(y.size());
for (int i = 0; i < y.size(); i++) {
b(i) = y[i];
}
// Solve the least squares problem
VectorXd coeffs = A.bdcSvd(ComputeThinU | ComputeThinV).solve(b);
// Evaluate the fitted function at some new points
std::vector<double> newX = {0.5, 1.5, 2.5, 3.5, 4.5};
std::vector<double> newY(newX.size());
for (int i = 0; i < newX.size(); i++) {
double value = 0.0;
for (int j = 0; j < degree + 1; j++) {
value += coeffs(j) * pow(newX[i], j);
}
newY[i] = value;
}
// Print the coefficients of the fitted polynomial
std::cout << "Coefficients: ";
for (int i = 0; i < degree + 1; i++) {
std::cout << coeffs(i) << " ";
}
std::cout << std::endl;
// Plot the original points and the fitted function
// (not implemented here)
// Compute the mean squared error between the fitted function and the original points
double mse = 0.0;
for (int i = 0; i < x.size(); i++) {
double value = 0.0;
for (int j = 0; j < degree + 1; j++) {
value += coeffs(j) * pow(x[i], j);
}
mse += pow(value - y[i], 2.0);
}
mse /= x.size();
std::cout << "Mean squared error: " << mse << std::endl;
return 0;
}
```
该示例代码使用多项式函数来拟合一些给定的点,并计算了误差。在实际应用中,可以根据需要选择不同的拟合函数。
阅读全文
相关推荐
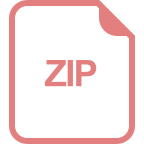


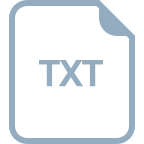
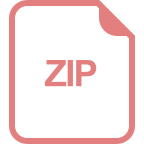
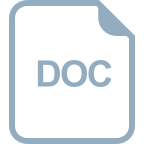
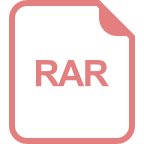
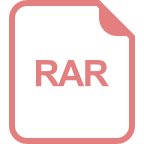
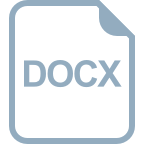
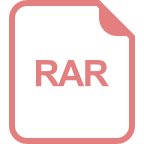
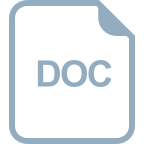
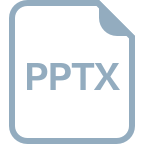
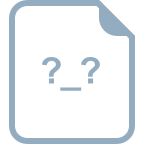
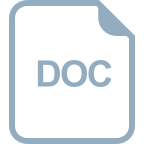
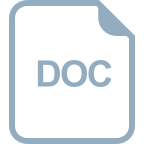
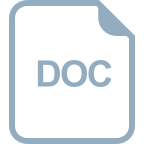
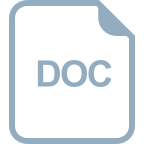