用libtiff如何将cmyk四张分色图合并成一张tiff,并保存一张bmp缩略图?
时间: 2024-05-05 20:17:11 浏览: 245
以下是使用libtiff库将CMYK四张分色图合并成一张TIFF,并保存一张BMP缩略图的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <tiffio.h>
int main(int argc, char** argv)
{
if (argc < 6) {
printf("Usage: %s c.tif m.tif y.tif k.tif out.tif out.bmp\n", argv[0]);
return 0;
}
// Open input files
TIFF* c_tiff = TIFFOpen(argv[1], "r");
TIFF* m_tiff = TIFFOpen(argv[2], "r");
TIFF* y_tiff = TIFFOpen(argv[3], "r");
TIFF* k_tiff = TIFFOpen(argv[4], "r");
if (!c_tiff || !m_tiff || !y_tiff || !k_tiff) {
printf("Error: Failed to open input files\n");
return 0;
}
// Get image width and height
uint32_t width, height;
TIFFGetField(c_tiff, TIFFTAG_IMAGEWIDTH, &width);
TIFFGetField(c_tiff, TIFFTAG_IMAGELENGTH, &height);
// Create output TIFF file
TIFF* out_tiff = TIFFOpen(argv[5], "w");
if (!out_tiff) {
printf("Error: Failed to create output TIFF file\n");
return 0;
}
// Set TIFF tags
TIFFSetField(out_tiff, TIFFTAG_IMAGEWIDTH, width);
TIFFSetField(out_tiff, TIFFTAG_IMAGELENGTH, height);
TIFFSetField(out_tiff, TIFFTAG_SAMPLESPERPIXEL, 4);
TIFFSetField(out_tiff, TIFFTAG_BITSPERSAMPLE, 8);
TIFFSetField(out_tiff, TIFFTAG_PHOTOMETRIC, PHOTOMETRIC_SEPARATED);
TIFFSetField(out_tiff, TIFFTAG_PLANARCONFIG, PLANARCONFIG_CONTIG);
TIFFSetField(out_tiff, TIFFTAG_ROWSPERSTRIP, TIFFDefaultStripSize(out_tiff, width * 4));
// Allocate memory for image data
uint8_t* data = (uint8_t*)malloc(width * height * 4 * sizeof(uint8_t));
if (!data) {
printf("Error: Failed to allocate memory for image data\n");
return 0;
}
// Read image data from input files
TIFFReadRGBAImage(c_tiff, width, height, (uint32_t*)data, 0);
TIFFReadRGBAImage(m_tiff, width, height, (uint32_t*)(data + width * height * 4), 0);
TIFFReadRGBAImage(y_tiff, width, height, (uint32_t*)(data + width * height * 8), 0);
TIFFReadRGBAImage(k_tiff, width, height, (uint32_t*)(data + width * height * 12), 0);
// Write image data to output file
TIFFWriteEncodedStrip(out_tiff, 0, data, width * height * 4);
// Create BMP thumbnail
TIFF* bmp_tiff = TIFFOpen(argv[6], "w");
if (!bmp_tiff) {
printf("Error: Failed to create BMP thumbnail\n");
return 0;
}
// Set TIFF tags for BMP
TIFFSetField(bmp_tiff, TIFFTAG_IMAGEWIDTH, width);
TIFFSetField(bmp_tiff, TIFFTAG_IMAGELENGTH, height);
TIFFSetField(bmp_tiff, TIFFTAG_PHOTOMETRIC, PHOTOMETRIC_RGB);
TIFFSetField(bmp_tiff, TIFFTAG_PLANARCONFIG, PLANARCONFIG_CONTIG);
TIFFSetField(bmp_tiff, TIFFTAG_BITSPERSAMPLE, 8);
TIFFSetField(bmp_tiff, TIFFTAG_SAMPLESPERPIXEL, 3);
TIFFSetField(bmp_tiff, TIFFTAG_ROWSPERSTRIP, height);
// Write BMP data
uint8_t* bmp_data = (uint8_t*)malloc(width * height * 3 * sizeof(uint8_t));
for (uint32_t i = 0; i < height; i++) {
for (uint32_t j = 0; j < width; j++) {
uint8_t* pixel = data + (i * width + j) * 4;
bmp_data[(i * width + j) * 3] = 255 - pixel[0];
bmp_data[(i * width + j) * 3 + 1] = 255 - pixel[1];
bmp_data[(i * width + j) * 3 + 2] = 255 - pixel[2];
}
}
TIFFWriteEncodedStrip(bmp_tiff, 0, bmp_data, width * height * 3);
// Close files and free memory
TIFFClose(c_tiff);
TIFFClose(m_tiff);
TIFFClose(y_tiff);
TIFFClose(k_tiff);
TIFFClose(out_tiff);
TIFFClose(bmp_tiff);
free(data);
free(bmp_data);
return 0;
}
```
该程序需要传入四个CMYK分色图的文件名,一个输出TIFF文件名和一个输出BMP缩略图的文件名。程序首先打开输入文件并获取图像的宽度和高度。然后,它创建一个新的TIFF文件并设置必要的标记,例如图像宽度,高度,样本数,位数和颜色模型。接下来,程序将分配足够的内存来存储图像数据,并从四个输入文件中读取RGBA数据。最后,程序将此数据写入输出TIFF文件和BMP缩略图。请注意,BMP缩略图是将CMYK颜色转换为RGB并反相的结果。程序成功完成后,它将关闭所有文件并释放分配的内存。
希望这可以帮助你!
阅读全文
相关推荐



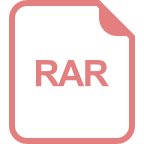
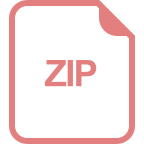
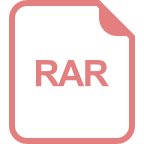
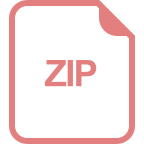
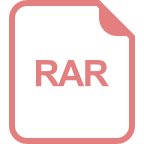
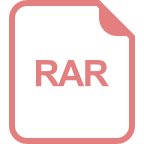
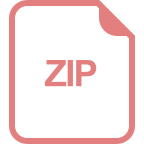
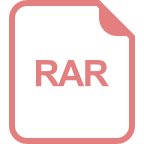
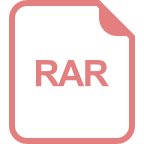



