基于C++,libtiff和OpenCV库把bmp图像通过金字塔结构转换成一张具有多个页的tif图像,可以提供代码吗
时间: 2023-11-22 16:56:37 浏览: 164
以下是基于C++,使用libtiff和OpenCV库将bmp图像转换成多页tif图像的示例代码:
```c++
#include <iostream>
#include <string>
#include <vector>
#include "opencv2/opencv.hpp"
#include "tiffio.h"
using namespace std;
using namespace cv;
void bmp2tiff(string input_image_path, string output_tiff_path)
{
// Load BMP image
Mat image = imread(input_image_path, IMREAD_GRAYSCALE);
if (image.empty())
{
cerr << "Failed to load image: " << input_image_path << endl;
return;
}
// Initialize TIFF file
TIFF* tiff = TIFFOpen(output_tiff_path.c_str(), "w");
if (tiff == NULL)
{
cerr << "Failed to create file: " << output_tiff_path << endl;
return;
}
// Set TIFF tags
TIFFSetField(tiff, TIFFTAG_IMAGEWIDTH, image.cols);
TIFFSetField(tiff, TIFFTAG_IMAGELENGTH, image.rows);
TIFFSetField(tiff, TIFFTAG_SAMPLESPERPIXEL, 1);
TIFFSetField(tiff, TIFFTAG_BITSPERSAMPLE, 8);
TIFFSetField(tiff, TIFFTAG_ORIENTATION, ORIENTATION_TOPLEFT);
TIFFSetField(tiff, TIFFTAG_COMPRESSION, COMPRESSION_NONE);
TIFFSetField(tiff, TIFFTAG_PHOTOMETRIC, PHOTOMETRIC_MINISBLACK);
TIFFSetField(tiff, TIFFTAG_ROWSPERSTRIP, image.rows);
// Write image data to TIFF file
TIFFWriteEncodedStrip(tiff, 0, image.data, image.cols * image.rows);
// Generate image pyramid and write to TIFF file as multiple pages
Mat pyramid = image;
vector<uchar> buffer;
int strip_size = TIFFStripSize(tiff);
for (int level = 1; pyramid.cols > 1 && pyramid.rows > 1; level++)
{
// Downsample image
pyrDown(pyramid, pyramid);
// Set TIFF tags for new page
TIFFSetField(tiff, TIFFTAG_SUBFILETYPE, FILETYPE_PAGE);
TIFFSetField(tiff, TIFFTAG_PAGENUMBER, level, level);
TIFFWriteDirectory(tiff);
// Write image data to TIFF file
buffer.resize(strip_size);
for (int y = 0; y < pyramid.rows; y += TIFFDefaultStripSize(tiff, NULL))
{
int rows_to_write = min(TIFFDefaultStripSize(tiff, y), pyramid.rows - y);
memcpy(&buffer[0], pyramid.ptr(y), rows_to_write * pyramid.step[0]);
TIFFWriteEncodedStrip(tiff, TIFFComputeStrip(tiff, y, 0), &buffer[0], rows_to_write * pyramid.step[0]);
}
}
// Close TIFF file
TIFFClose(tiff);
}
int main()
{
// Convert BMP to TIFF with pyramid structure
bmp2tiff("input.bmp", "output.tif");
return 0;
}
```
该代码使用OpenCV库将BMP图像加载到Mat对象中,然后使用TIFF库创建并初始化多页TIFF文件。接着,代码使用OpenCV库生成图像金字塔,并将每个级别的图像作为TIFF文件的一页进行写入。最后,代码关闭TIFF文件并返回。
请注意,由于金字塔结构会生成多个图像,因此我们需要在TIFF文件中创建多个页面。通过设置TIFF标签`TIFFTAG_SUBFILETYPE`和`TIFFTAG_PAGENUMBER`,我们可以为每个页面指定类型和页码。在写入每个页面的图像数据之前,我们需要调用`TIFFWriteDirectory`函数,以便TIFF库知道我们要写入新的页面。
此外,由于`TIFFWriteEncodedStrip`函数可以一次写入一条条带,因此我们需要计算条带的大小,并将图像数据分成多个条带进行写入。在此示例代码中,我们使用`TIFFDefaultStripSize`函数计算每个条带的默认大小,并在循环中一次性写入多个条带。
阅读全文
相关推荐
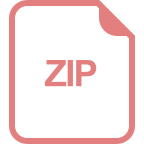
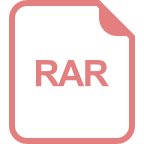
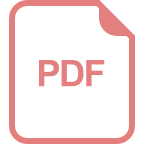
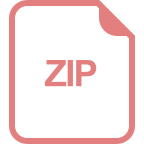
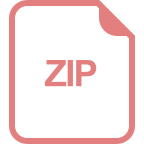
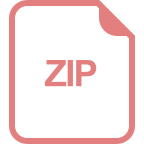
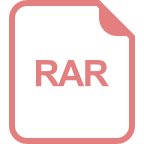
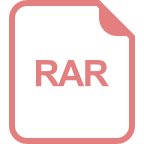
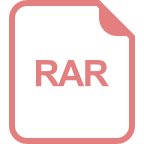








