interface Printable { public abstract float sum(float x ,float y); } class AAA implements Printable { /* 请在这里填写答案 */ } public class Main { public static void main(String args[]) { AAA a = new AAA(); System.out.println("调用sum方法(重写的):"+a.sum(12,18)); } }
时间: 2024-03-25 17:40:50 浏览: 16
好的,根据题意,需要在AAA类中对接口Printable中的sum方法进行重写。可以参考如下代码:
```java
interface Printable {
public abstract float sum(float x, float y);
}
class AAA implements Printable {
@Override
public float sum(float x, float y) {
return x + y;
}
}
public class Main {
public static void main(String args[]) {
AAA a = new AAA();
System.out.println("调用sum方法(重写的):" + a.sum(12, 18));
}
}
```
在这个实现中,我们首先定义了接口Printable,其中包含一个抽象方法sum(float x, float y)。接着,我们定义了一个AAA类,该类实现了Printable接口,并对sum方法进行了重写,使其可以对两个float类型的参数进行求和操作。最后,在Main类中,我们创建了一个AAA对象,并调用了其重写后的sum方法,输出其结果。
相关问题
pta接口实现请实现接口Printable中的sum方法。
好的,我来给你实现一个pta接口的Printable中的sum方法的样例代码:
```java
public class PTA implements Printable {
private int[] arr;
public PTA(int[] arr) {
this.arr = arr;
}
@Override
public int sum() {
int sum = 0;
for (int i : arr) {
sum += i;
}
return sum;
}
//其他方法的实现
}
```
在这个实现中,我们首先定义了一个PTA类,该类实现了Printable接口,并且具有一个整型数组arr作为它的成员变量。接着,在sum()方法中,我们遍历整型数组arr,并将数组中的所有元素相加,最后返回它们的和。
interface class
接口类(Interface Class)是一种特殊类型的类,它只包含方法的声明而没有方法的实现。它定义了一组方法的契约,任何实现该接口的类都必须提供这些方法的具体实现。接口类在面向对象编程中被用来实现多态性和代码重用。
接口类使用关键字 "interface" 来定义,其语法如下:
```
public interface InterfaceName {
// 方法声明
}
```
接口类中的方法默认为公有(public),不需要显式地声明为公有。实现接口类的类必须提供接口中所有方法的具体实现。
例如,下面是一个简单的接口类和一个实现了该接口的类的示例:
```java
public interface Printable {
void print();
}
public class Printer implements Printable {
public void print() {
System.out.println("Printing...");
}
}
```
在上面的示例中,Printable 是一个接口类,它定义了一个名为 print 的方法。Printer 是一个实现了 Printable 接口的类,它提供了 print 方法的具体实现。
通过使用接口类,我们可以实现多个类之间的代码重用,并通过多态性来替换具体实现。
相关推荐
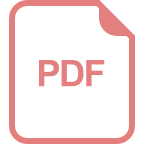
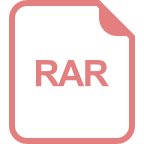












