使用python将多类型相机根据不同视野、工作距离、帧率、精度通过3D图的形式显示出来
时间: 2023-08-03 14:04:02 浏览: 41
要实现这个需求,需要用到以下工具和库:
- OpenCV:用于处理图像和视频;
- Matplotlib:用于绘制3D图形;
- NumPy:用于数据处理和计算。
步骤如下:
1. 读取不同类型相机的视频流,并将每一帧转换为3D坐标系中的点。
2. 根据每个相机的视野、工作距离和帧率,计算出每个点的位置和颜色。
3. 将所有点绘制在3D图形上,并添加坐标轴和标题等元素。
代码示例:
```python
import cv2
import numpy as np
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
# 读取不同类型相机的视频流
cam1 = cv2.VideoCapture(0)
cam2 = cv2.VideoCapture(1)
# 设置相机参数
fov1 = 60 # 视野角度
fov2 = 45
distance1 = 1 # 工作距离
distance2 = 2
fps1 = 30 # 帧率
fps2 = 60
# 创建3D图形
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
ax.set_title('Multi-camera 3D Scene')
# 循环读取视频流
while True:
# 读取每个相机的一帧
ret1, frame1 = cam1.read()
ret2, frame2 = cam2.read()
if not ret1 or not ret2:
break
# 将每一帧转换为3D坐标系中的点
points1 = []
points2 = []
for y in range(frame1.shape[0]):
for x in range(frame1.shape[1]):
# 计算像素点在3D坐标系中的位置
z1 = distance1 * np.tan(np.deg2rad(fov1 / 2)) * 2 / frame1.shape[1] * (x - frame1.shape[1] / 2)
y1 = -distance1 * np.tan(np.deg2rad(fov1 / 2)) * 2 / frame1.shape[0] * (y - frame1.shape[0] / 2)
x1 = distance1
z2 = distance2 * np.tan(np.deg2rad(fov2 / 2)) * 2 / frame2.shape[1] * (x - frame2.shape[1] / 2)
y2 = -distance2 * np.tan(np.deg2rad(fov2 / 2)) * 2 / frame2.shape[0] * (y - frame2.shape[0] / 2)
x2 = distance2
# 计算像素点的颜色
color1 = frame1[y, x].tolist()
color2 = frame2[y, x].tolist()
# 添加点到列表中
points1.append([x1, y1, z1, color1])
points2.append([x2, y2, z2, color2])
# 将所有点绘制在3D图形上
xs1, ys1, zs1, colors1 = zip(*points1)
xs2, ys2, zs2, colors2 = zip(*points2)
ax.scatter(xs1, ys1, zs1, c=colors1)
ax.scatter(xs2, ys2, zs2, c=colors2)
# 显示3D图形
plt.pause(1 / fps1)
# 关闭相机和窗口
cam1.release()
cam2.release()
plt.close()
```
运行以上代码,即可看到多类型相机拍摄的3D场景。根据需要,可以调整相机参数和3D图形的样式。
相关推荐
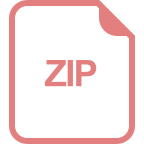
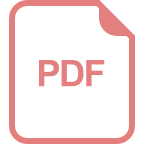














