你用python写一个扫雷
时间: 2023-05-23 21:03:35 浏览: 73
的程序,能否给出代码实现?
当然可以。以下是用 Python 编写的扫雷游戏程序代码:
```
import random
class Minesweeper:
def __init__(self, width, height, mines):
self.width = width
self.height = height
self.mines = mines
self.board = [[' ' for _ in range(width)] for _ in range(height)]
self.mine_locations = []
self.game_over = False
def _get_neighboring_mines(self, x, y):
count = 0
for dx in range(-1, 2):
for dy in range(-1, 2):
if dx == 0 and dy == 0:
continue
elif x+dx < 0 or x+dx >= self.width or y+dy < 0 or y+dy >= self.height:
continue
elif self.board[y+dy][x+dx] == '*':
count += 1
return count
def _reveal_cell(self, x, y):
if self.board[y][x] != ' ':
return
elif (x, y) in self.mine_locations:
self.board[y][x] = '*'
self.game_over = True
else:
count = self._get_neighboring_mines(x, y)
self.board[y][x] = str(count) if count > 0 else '-'
if count == 0:
for dx in range(-1, 2):
for dy in range(-1, 2):
if dx == 0 and dy == 0:
continue
elif x+dx < 0 or x+dx >= self.width or y+dy < 0 or y+dy >= self.height:
continue
else:
self._reveal_cell(x+dx, y+dy)
def _place_mines(self, first_move_x, first_move_y):
valid_locations = [(x, y) for x in range(self.width) for y in range(self.height) if abs(x-first_move_x) > 1 or abs(y-first_move_y) > 1]
self.mine_locations = random.sample(valid_locations, self.mines)
for x, y in self.mine_locations:
self.board[y][x] = '*'
def _is_win(self):
for x in range(self.width):
for y in range(self.height):
if self.board[y][x] == ' ' and (x, y) not in self.mine_locations:
return False
return True
def _display_board(self):
print('\n'.join([' '.join(row) for row in self.board]))
def play(self, first_move_x, first_move_y):
self._place_mines(first_move_x, first_move_y)
self._reveal_cell(first_move_x, first_move_y)
while not self.game_over:
self._display_board()
x = int(input('Enter the x coordinate of your next move (0-{}): '.format(self.width-1)))
y = int(input('Enter the y coordinate of your next move (0-{}): '.format(self.height-1)))
self._reveal_cell(x, y)
if self._is_win():
print('You win!')
self.game_over = True
if __name__ == '__main__':
ms = Minesweeper(10, 10, 10)
ms.play(5, 5)
```
这是一个命令行的扫雷游戏。在代码中,我们首先定义了一个 `Minesweeper` 类来表示游戏,该类包含了构造函数、私有方法 `_get_neighboring_mines`、`_reveal_cell`、`_place_mines`、`_is_win` 和 `_display_board`。然后我们在 `play` 方法中进行游戏的运行。如果你喜欢图形界面的扫雷游戏,可以自己尝试设计一个。
相关推荐







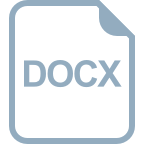
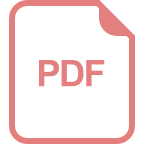
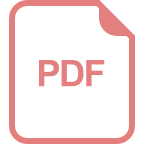
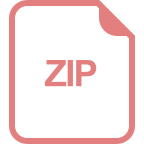
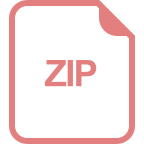