用Python写一个扫雷
时间: 2023-12-29 11:17:15 浏览: 124
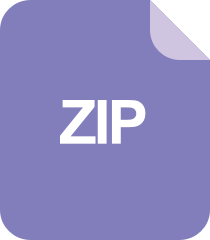
基于Python(Tkinter)实现的扫雷游戏
好的,以下是一个简单的扫雷游戏示例代码:
```python
import random
# 初始化游戏地图
def init_map(rows, cols, mines):
# 创建一个 rows 行,cols 列,全部填充为 0 的二维数组
map = [[0 for j in range(cols)] for i in range(rows)]
# 在地图上随机布置 mines 个地雷
for i in range(mines):
row = random.randint(0, rows-1)
col = random.randint(0, cols-1)
# 如果当前位置已经有地雷,则重新选择位置
while map[row][col] == -1:
row = random.randint(0, rows-1)
col = random.randint(0, cols-1)
map[row][col] = -1
return map
# 计算当前位置周围地雷数量
def count_mines(map, row, col):
count = 0
for i in range(row-1, row+2):
for j in range(col-1, col+2):
if i >= 0 and i < len(map) and j >= 0 and j < len(map[0]) and map[i][j] == -1:
count += 1
return count
# 显示游戏地图
def display_map(map, show_all=False):
for row in map:
for col in row:
if show_all or col >= 0:
print(col if col >= 0 else '*', end=' ')
else:
print('.', end=' ')
print()
# 主函数
def main():
# 游戏参数
rows = 10
cols = 10
mines = 10
# 初始化游戏地图
map = init_map(rows, cols, mines)
# 开始游戏
while True:
# 显示当前地图
display_map(map)
# 获取用户输入
row = int(input('请输入行号:'))
col = int(input('请输入列号:'))
# 判断用户选择的位置是否有地雷
if map[row][col] == -1:
print('你踩到地雷了,游戏结束!')
display_map(map, True)
break
# 计算当前位置周围地雷数量,并更新地图
count = count_mines(map, row, col)
map[row][col] = count
# 判断是否已经胜利
if sum(row.count(-1) for row in map) == mines:
print('恭喜你,你赢了!')
display_map(map, True)
break
if __name__ == '__main__':
main()
```
这个示例代码实现了一个简单的扫雷游戏,游戏参数可以自行调整。在游戏中,玩家需要输入行号和列号来选择一个方格,如果选择的方格上有地雷,游戏失败;如果选择的方格周围有地雷,则显示地雷数量;如果选择的方格周围没有地雷,则递归地展开周围空白区域,直到边界或有地雷为止。如果所有地雷都被找出,游戏胜利。
阅读全文
相关推荐
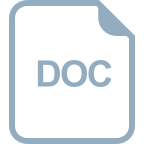
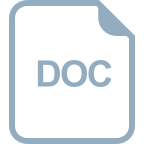





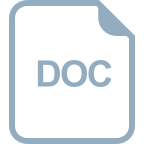
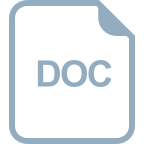
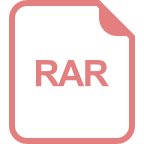
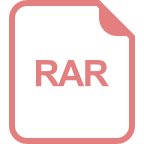
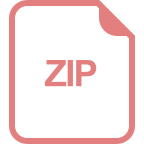
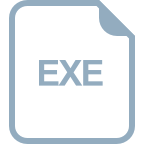
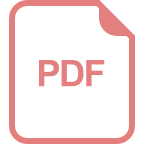