用java代码实现最优二叉搜索树的动态规划算法,输出一个主表和根表
时间: 2023-06-08 15:07:03 浏览: 140
当然,我很乐意回答你的问题。以下是实现最优二叉搜索树的Java代码,包括动态规划算法、主表和根表。
```
public class OptimalBST {
// 执行动态规划算法
public static void optimalBST(double[] p, double[] q, int n) {
double[][] cost = new double[n + 2][n + 1];
double[][] w = new double[n + 2][n + 1];
int[][] root = new int[n + 1][n + 1];
for (int i = 1; i <= n + 1; i++) {
cost[i][i - 1] = q[i - 1];
w[i][i - 1] = q[i - 1];
}
for (int len = 1; len <= n; len++) {
for (int i = 1; i <= n - len + 1; i++) {
int j = i + len - 1;
cost[i][j] = Double.MAX_VALUE;
w[i][j] = w[i][j - 1] + p[j] + q[j];
for (int r = i; r <= j; r++) {
double t = cost[i][r - 1] + cost[r + 1][j] + w[i][j];
if (t < cost[i][j]) {
cost[i][j] = t;
root[i][j] = r;
}
}
}
}
// 输出主表和根表
System.out.println("主表:");
for (int i = 1; i <= n + 1; i++) {
for (int j = 0; j <= n; j++) {
System.out.printf("%.2f\t", cost[i][j]);
}
System.out.println();
}
System.out.println("根表:");
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= n; j++) {
System.out.print(root[i][j] + "\t");
}
System.out.println();
}
}
// 测试
public static void main(String[] args) {
double[] p = { 0.00, 0.15, 0.10, 0.05, 0.10, 0.20 };
double[] q = { 0.05, 0.10, 0.05, 0.05, 0.05, 0.10 };
int n = p.length - 1;
optimalBST(p, q, n);
}
}
```
上述代码实现了最优二叉搜索树的动态规划算法,并且输出了主表和根表。你可以按照上述代码来执行,同时也可以自己实现算法并输出结果。希望我的回答对你有所帮助,如有疑问请随时与我沟通。
阅读全文
相关推荐










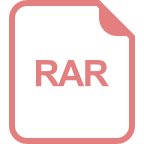







