使用pipe函数写一段c语言代码
时间: 2024-05-04 11:21:39 浏览: 15
以下是使用pipe函数创建父子进程间通信的简单示例代码:
```c
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <string.h>
#define BUFFER_SIZE 512
int main() {
int pipefd[2];
pid_t pid;
char buffer[BUFFER_SIZE];
// 创建管道
if (pipe(pipefd) == -1) {
perror("pipe");
exit(EXIT_FAILURE);
}
// 创建子进程
pid = fork();
if (pid == -1) {
perror("fork");
exit(EXIT_FAILURE);
}
// 父进程
if (pid > 0) {
// 关闭读端,向子进程写入数据
close(pipefd[0]);
printf("Enter a message: ");
fgets(buffer, BUFFER_SIZE, stdin);
write(pipefd[1], buffer, strlen(buffer));
close(pipefd[1]);
exit(EXIT_SUCCESS);
}
// 子进程
else {
// 关闭写端,从父进程读取数据
close(pipefd[1]);
read(pipefd[0], buffer, BUFFER_SIZE);
printf("Received message: %s", buffer);
close(pipefd[0]);
exit(EXIT_SUCCESS);
}
}
```
该程序会创建一个管道,然后创建一个子进程。在父进程中,它会从标准输入读取一个消息,并将其写入管道中,然后退出。在子进程中,它会从管道中读取消息,并将其输出到屏幕上,然后退出。注意,在使用管道时,必须确保关闭不需要的文件描述符,否则可能会导致死锁等问题。
相关推荐
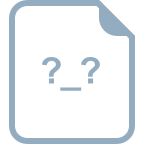
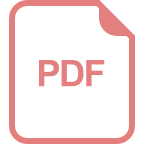














