sqlserver string operation
时间: 2023-08-21 21:04:07 浏览: 80
SQL Server 中有很多字符串操作函数,以下是一些常用的函数:
1. LEFT(string, length):返回字符串 string 最左边的 length 个字符。
2. RIGHT(string, length):返回字符串 string 最右边的 length 个字符。
3. SUBSTRING(string, start, length):返回字符串 string 从 start 开始的 length 个字符。
4. LEN(string):返回字符串 string 的长度。
5. REPLACE(string, old_string, new_string):将字符串 string 中的 old_string 替换为 new_string。
6. UPPER(string):将字符串 string 转换为大写。
7. LOWER(string):将字符串 string 转换为小写。
8. LTRIM(string):去除字符串 string 左边的空格。
9. RTRIM(string):去除字符串 string 右边的空格。
10. CHARINDEX(substring, string):返回子字符串 substring 在字符串 string 中的位置。
这些函数可以用于 SQL Server 中的 SELECT 语句,如:
SELECT LEFT('Hello World', 5)
返回结果为 "Hello"。
相关问题
学生选课管理系统javaweb,sqlserver代码实现
以下是一个简单的学生选课管理系统的JavaWeb代码,使用SQL Server作为数据库。本代码实现了学生登录、选课、查看选课情况等功能。请注意该代码仅供参考。
1.数据库设计
学生表(Student)
- ID(学生ID)
- Name(学生姓名)
- Password(学生密码)
课程表(Course)
- ID(课程ID)
- Name(课程名称)
- Teacher(任课教师)
- Credit(学分)
选课表(Selection)
- ID(选课ID)
- StudentID(学生ID)
- CourseID(课程ID)
2.登录页面(index.jsp)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生选课管理系统</title>
</head>
<body>
<h1>学生选课管理系统</h1>
<form action="login" method="post">
<label for="id">学号:</label>
<input type="text" id="id" name="id"><br>
<label for="password">密码:</label>
<input type="password" id="password" name="password"><br>
<input type="submit" value="登录">
</form>
</body>
</html>
3.登录处理(LoginServlet.java)
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
@WebServlet("/login")
public class LoginServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
String id = request.getParameter("id");
String password = request.getParameter("password");
String message = "";
try {
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
String url = "jdbc:sqlserver://localhost:1433;databaseName=StudentCourse";
String user = "sa";
String pass = "123456";
Connection con = DriverManager.getConnection(url, user, pass);
String sql = "SELECT * FROM Student WHERE ID=? AND Password=?";
PreparedStatement pstmt = con.prepareStatement(sql);
pstmt.setString(1, id);
pstmt.setString(2, password);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
HttpSession session = request.getSession(true);
session.setAttribute("id", id);
response.sendRedirect("course");
return;
} else {
message = "学号或密码错误";
}
rs.close();
pstmt.close();
con.close();
} catch (ClassNotFoundException e) {
message = "数据库驱动程序未找到";
e.printStackTrace();
} catch (SQLException e) {
message = "数据库操作异常";
e.printStackTrace();
}
request.setAttribute("message", message);
request.getRequestDispatcher("index.jsp").forward(request, response);
}
}
4.选课页面(course.jsp)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生选课管理系统</title>
</head>
<body>
<h1>学生选课管理系统</h1>
<h2>欢迎,<%=session.getAttribute("id")%></h2>
<h3>当前选课情况:</h3>
<table>
<tr>
<th>课程编号</th>
<th>课程名称</th>
<th>任课教师</th>
<th>学分</th>
<th>操作</th>
</tr>
<%@ page import="java.util.*" %>
<%@ page import="java.sql.*" %>
<%
String id = (String)session.getAttribute("id");
String message = "";
try {
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
String url = "jdbc:sqlserver://localhost:1433;databaseName=StudentCourse";
String user = "sa";
String pass = "123456";
Connection con = DriverManager.getConnection(url, user, pass);
String sql1 = "SELECT * FROM Selection WHERE StudentID=?";
PreparedStatement pstmt1 = con.prepareStatement(sql1);
pstmt1.setString(1, id);
ResultSet rs1 = pstmt1.executeQuery();
List<String> courses = new ArrayList<String>();
while (rs1.next()) {
courses.add(rs1.getString("CourseID"));
}
rs1.close();
pstmt1.close();
String sql2 = "SELECT * FROM Course";
PreparedStatement pstmt2 = con.prepareStatement(sql2);
ResultSet rs2 = pstmt2.executeQuery();
while (rs2.next()) {
String courseID = rs2.getString("ID");
String courseName = rs2.getString("Name");
String teacher = rs2.getString("Teacher");
String credit = rs2.getString("Credit");
String operation = "";
if (courses.contains(courseID)) {
operation = "<a href='drop?id=" + courseID + "'>退选</a>";
} else {
operation = "<a href='choose?id=" + courseID + "'>选课</a>";
}
out.print("<tr><td>" + courseID + "</td><td>" + courseName + "</td><td>" + teacher + "</td><td>" + credit + "</td><td>" + operation + "</td></tr>");
}
rs2.close();
pstmt2.close();
con.close();
} catch (ClassNotFoundException e) {
message = "数据库驱动程序未找到";
e.printStackTrace();
} catch (SQLException e) {
message = "数据库操作异常";
e.printStackTrace();
}
%>
</table>
<p><%=message%></p>
</body>
</html>
5.选课处理(ChooseServlet.java)
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
@WebServlet("/choose")
public class ChooseServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
HttpSession session = request.getSession(true);
String id = (String)session.getAttribute("id");
String courseID = request.getParameter("id");
String message = "";
try {
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
String url = "jdbc:sqlserver://localhost:1433;databaseName=StudentCourse";
String user = "sa";
String pass = "123456";
Connection con = DriverManager.getConnection(url, user, pass);
String sql = "INSERT INTO Selection(StudentID, CourseID) VALUES(?, ?)";
PreparedStatement pstmt = con.prepareStatement(sql);
pstmt.setString(1, id);
pstmt.setString(2, courseID);
pstmt.executeUpdate();
pstmt.close();
con.close();
} catch (ClassNotFoundException e) {
message = "数据库驱动程序未找到";
e.printStackTrace();
} catch (SQLException e) {
message = "数据库操作异常";
e.printStackTrace();
}
request.setAttribute("message", message);
request.getRequestDispatcher("course.jsp").forward(request, response);
}
}
6.退选处理(DropServlet.java)
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
@WebServlet("/drop")
public class DropServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
HttpSession session = request.getSession(true);
String id = (String)session.getAttribute("id");
String courseID = request.getParameter("id");
String message = "";
try {
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
String url = "jdbc:sqlserver://localhost:1433;databaseName=StudentCourse";
String user = "sa";
String pass = "123456";
Connection con = DriverManager.getConnection(url, user, pass);
String sql = "DELETE FROM Selection WHERE StudentID=? AND CourseID=?";
PreparedStatement pstmt = con.prepareStatement(sql);
pstmt.setString(1, id);
pstmt.setString(2, courseID);
pstmt.executeUpdate();
pstmt.close();
con.close();
} catch (ClassNotFoundException e) {
message = "数据库驱动程序未找到";
e.printStackTrace();
} catch (SQLException e) {
message = "数据库操作异常";
e.printStackTrace();
}
request.setAttribute("message", message);
request.getRequestDispatcher("course.jsp").forward(request, response);
}
}
注意:本代码仅供参考,请不要直接用于实际应用中,需要根据实际情况进行修改和完善。
fail: 2023/7/14 14:31:33.417 CoreEventId.QueryIterationFailed[10100] (Microsoft.EntityFrameworkCore.Query) An exception occurred while iterating over the results of a query for context type 'iMES.Core.EFDbContext.SysDbContext'. System.InvalidOperationException: There is already an open DataReader associated with this Connection which must be closed first. at Microsoft.Data.SqlClient.SqlInternalConnectionTds.ValidateConnectionForExecute(SqlCommand command) at Microsoft.Data.SqlClient.SqlConnection.ValidateConnectionForExecute(String method, SqlCommand command) at Microsoft.Data.SqlClient.SqlCommand.ValidateCommand(Boolean isAsync, String method) at Microsoft.Data.SqlClient.SqlCommand.RunExecuteReader(CommandBehavior cmdBehavior, RunBehavior runBehavior, Boolean returnStream, TaskCompletionSource`1 completion, Int32 timeout, Task& task, Boolean& usedCache, Boolean asyncWrite, Boolean inRetry, String method) at Microsoft.Data.SqlClient.SqlCommand.RunExecuteReader(CommandBehavior cmdBehavior, RunBehavior runBehavior, Boolean returnStream, String method) at Microsoft.Data.SqlClient.SqlCommand.ExecuteReader(CommandBehavior behavior) at Microsoft.Data.SqlClient.SqlCommand.ExecuteDbDataReader(CommandBehavior behavior) at Microsoft.EntityFrameworkCore.Storage.RelationalCommand.ExecuteReader(RelationalCommandParameterObject parameterObject) at Microsoft.EntityFrameworkCore.Query.Internal.SingleQueryingEnumerable`1.Enumerator.InitializeReader(Enumerator enumerator) at Microsoft.EntityFrameworkCore.Query.Internal.SingleQueryingEnumerable`1.Enumerator.<>c.<MoveNext>b__21_0(DbContext _, Enumerator enumerator) at Microsoft.EntityFrameworkCore.SqlServer.Storage.Internal.SqlServerExecutionStrategy.Execute[TState,TResult](TState state, Func`3 operation, Func`3 verifySucceeded) at Microsoft.EntityFrameworkCore.Query.Internal.SingleQueryingEnumerable`1.Enumerator.MoveNext()
根据您提供的信息,出现了一个异常,提示已经有一个与该连接关联的打开的 DataReader,必须先关闭它。
这个问题通常是由于在使用 Entity Framework 进行查询时,存在未关闭的 DataReader 导致的。DataReaders 是用于读取数据库查询结果的对象,在使用完毕后需要显式地关闭。
要解决这个问题,您可以尝试以下几点:
1. 确保在使用完 DataReader 后,及时调用其 Close() 或 Dispose() 方法来关闭它。可以使用 using 语句来自动处理这个操作,确保 DataReader 在使用完毕后被正确关闭。
2. 检查代码中是否存在嵌套的查询或循环查询,确保每个查询都正确关闭其对应的 DataReader。
3. 如果您的代码中使用了多个数据库连接对象,确保每个连接对象都能正确地关闭和释放资源。
4. 如果您使用的是异步查询,需要注意在异步操作完成后关闭 DataReader。
需要注意的是,以上建议是一般情况下解决该异常的方法。根据具体的代码和业务逻辑,您可能需要进一步检查和调整代码以确保 DataReader 的正确关闭。
希望以上建议对解决您的问题有所帮助!如果您需要更详细的帮助,请提供更多关于您的代码和查询的信息。
相关推荐
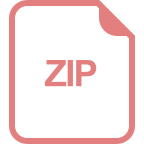
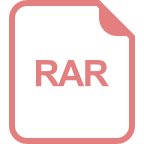
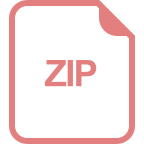









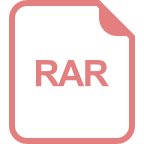
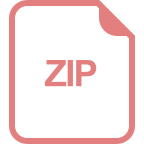
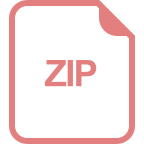
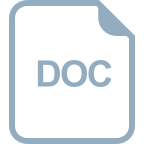