举例实现与SQL Server数据库的连接并进行增删操作
时间: 2024-10-20 19:09:05 浏览: 16
在C#中,我们可以使用`System.Data.SqlClient`命名空间下的类来连接到SQL Server数据库并执行CRUD(Create, Read, Update, Delete)操作。这里是一个简单的例子:
```csharp
using System;
using System.Data.SqlClient;
public class DatabaseExample
{
private string connectionString = "Data Source=your_server;Initial Catalog=your_database;User ID=your_username;Password=your_password;"; // 替换为实际的数据库连接信息
public void ConnectAndInsert()
{
using (SqlConnection connection = new SqlConnection(connectionString))
{
try
{
connection.Open();
string sqlInsert = "INSERT INTO YourTable (Column1, Column2) VALUES (@Value1, @Value2)";
SqlCommand command = new SqlCommand(sqlInsert, connection);
command.Parameters.AddWithValue("@Value1", "Value1");
command.Parameters.AddWithValue("@Value2", "Value2");
command.ExecuteNonQuery();
Console.WriteLine("Insert operation successful.");
}
catch (Exception ex)
{
Console.WriteLine($"Error occurred: {ex.Message}");
}
}
}
public void ConnectAndDelete(int id)
{
using (SqlConnection connection = new SqlConnection(connectionString))
{
try
{
connection.Open();
string sqlDelete = "DELETE FROM YourTable WHERE Id = @Id";
SqlCommand command = new SqlCommand(sqlDelete, connection);
command.Parameters.AddWithValue("@Id", id);
int rowsAffected = command.ExecuteNonQuery();
if (rowsAffected > 0)
Console.WriteLine($"Row with ID {id} deleted successfully.");
else
Console.WriteLine($"No row found with ID {id}.");
}
catch (Exception ex)
{
Console.WriteLine($"Error occurred: {ex.Message}");
}
}
}
}
```
在这个例子中,`ConnectAndInsert`方法插入新的记录,而`ConnectAndDelete`删除指定ID的记录。记得替换`YourTable`, `Column1`, `Column2`, `your_server`, `your_database`, `your_username`, 和 `your_password`为实际的表名、列名以及数据库连接配置。
阅读全文
相关推荐
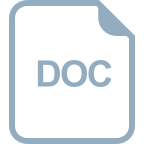
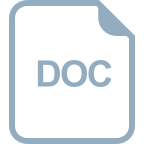
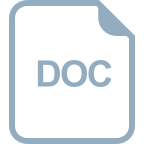

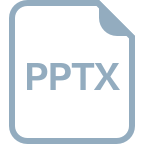
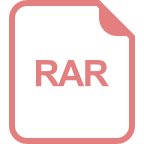
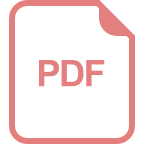
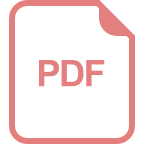
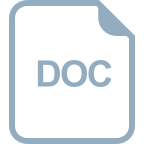
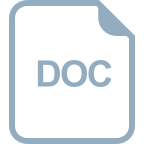
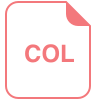




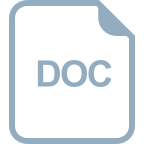
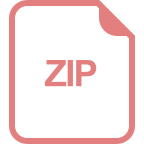
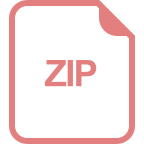
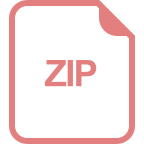