不知道python爬虫requests的headers怎么写怎么办
时间: 2023-06-25 15:02:58 浏览: 204
在使用 Python 爬虫 requests 发送请求时,headers 是一个非常重要的参数,可以用来传递一些请求头信息,比如 User-Agent、Referer、Cookies 等。可以按照以下格式设置 headers:
```python
import requests
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3',
'Referer': 'https://www.baidu.com/',
'Cookie': 'your_cookie_here',
}
response = requests.get('http://example.com', headers=headers)
```
其中,`User-Agent` 是浏览器请求头,`Referer` 是请求来源,`Cookie` 是请求携带的 Cookie。你可以根据自己的需要添加或修改这些请求头信息。
相关问题
python爬虫豆瓣headers
要爬取豆瓣网站的数据,你需要设置合适的 来模拟浏览器发送请求。下面是一个示例的 Python 爬虫代码,可以帮助你添加合适的 headers:
```python
import requests
# 设置请求头信息
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0;Win64) AppleWebkit/537.36 (KHTML,like Gecko) Chrome/89.0.4389.82 Safari/537.36'
}
# 发送 GET 请求并获取网页内容
url = 'https://www.douban.com/'
response = requests.get(url, headers=headers)
# 打印网页内容
print(response.text)
```
在上面的代码中,我们使用了 `requests` 库发送 GET 请求,并在请求中添加了一个名为 `User-Agent` 的 header。这个 header 用来告诉豆瓣服务器我们的请求是从一个浏览器发出的,而不是一个爬虫程序。你可以根据需要修改 `User-Agent` 的值。
此外,还有其他关键的 headers 信息可以添加,例如 `Referer` 和 `Cookie`。这些 headers 可能会根据你要访问的具体页面而有所变化。请确保你了解并遵守豆瓣网站的爬取规则,并适当设置请求头信息。
python爬虫requests步骤
使用 Python 编写爬虫通常需要用到 requests 库,以下是使用 requests 库进行爬虫的基本步骤:
1. 导入 requests 库
```python
import requests
```
2. 发送请求
使用 requests 库的 get() 或 post() 方法发送请求,传入目标网址作为参数。例如:
```python
response = requests.get('http://www.example.com')
```
3. 处理响应
获得响应后,可以通过 response 对象的属性和方法来获取响应信息。例如:
```python
# 获取响应状态码
status_code = response.status_code
# 获取响应内容
content = response.content
# 获取响应头
headers = response.headers
# 获取 Cookies
cookies = response.cookies
```
4. 解析响应
通常我们需要对响应进行解析,获取有用的数据。使用 BeautifulSoup 或 lxml 库可以方便地进行 HTML 解析,使用 json 库可以解析 JSON 数据。例如:
```python
# 使用 BeautifulSoup 解析 HTML
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.content, 'html.parser')
title = soup.title.text
# 使用 json 解析 JSON 数据
import json
data = json.loads(response.content)
```
5. 处理异常
在请求过程中可能会出现各种异常,例如网络连接异常、服务器返回错误等。使用 try-except 语句可以处理这些异常。例如:
```python
try:
response = requests.get('http://www.example.com')
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(e)
```
以上是使用 requests 库进行爬虫的基本步骤,具体使用时需要根据实际情况进行调整和补充。
阅读全文
相关推荐
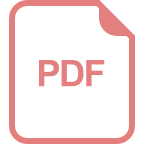
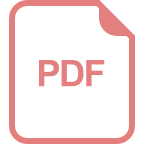
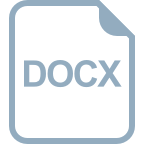
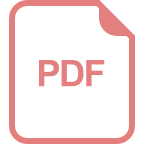
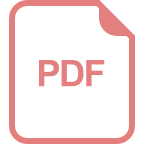










