常用C语言与汇编语言之间的对应关系:if else;switch;条件表达式;while;for等
时间: 2023-11-27 22:51:19 浏览: 74
下面是常用C语言与汇编语言之间的对应关系:
1. if else:
C语言:
```c
if (condition) {
// do something
}
else {
// do something else
}
```
汇编语言:
```asm
; compare the condition
cmp condition, 0
; jump if the condition is false
je else_branch
; do something if the condition is true
; ...
jmp end_if
else_branch:
; do something else if the condition is false
; ...
end_if:
```
2. switch:
C语言:
```c
switch (expression) {
case value1:
// do something
break;
case value2:
// do something else
break;
default:
// do something if none of the above cases match
break;
}
```
汇编语言:
```asm
; compare the expression to value1
cmp expression, value1
; jump to label1 if equal
je label1
; compare the expression to value2
cmp expression, value2
; jump to label2 if equal
je label2
; jump to default_label if none of the above cases match
jmp default_label
label1:
; do something for case value1
; ...
jmp end_switch
label2:
; do something for case value2
; ...
jmp end_switch
default_label:
; do something for default case
; ...
end_switch:
```
3. 条件表达式:
C语言:
```c
result = condition ? true_value : false_value;
```
汇编语言:
```asm
; compare the condition
cmp condition, 0
; jump to else_label if false
je else_label
; move true_value to result
mov result, true_value
; jump to end_if_label
jmp end_if_label
else_label:
; move false_value to result
mov result, false_value
end_if_label:
```
4. while:
C语言:
```c
while (condition) {
// do something
}
```
汇编语言:
```asm
while_loop:
; compare the condition
cmp condition, 0
; jump out of the loop if false
je end_while
; do something in the loop
; ...
; jump back to the top of the loop
jmp while_loop
end_while:
```
5. for:
C语言:
```c
for (i = 0; i < count; i++) {
// do something
}
```
汇编语言:
```asm
; initialize i to 0
mov i, 0
for_loop:
; compare i to count
cmp i, count
; jump out of the loop if i >= count
jge end_for
; do something in the loop
; ...
; increment i
inc i
; jump back to the top of the loop
jmp for_loop
end_for:
```
阅读全文
相关推荐
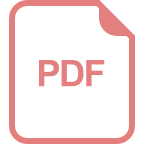

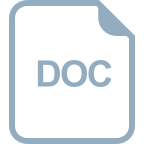
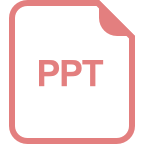
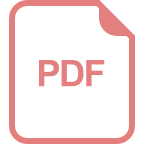
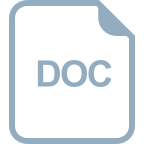
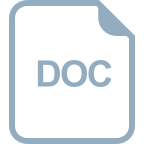
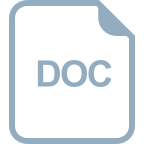
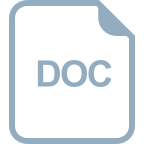
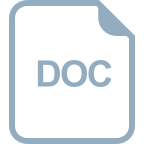
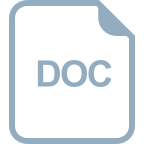
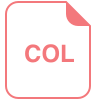
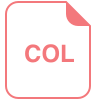
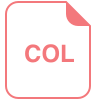
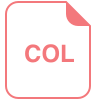
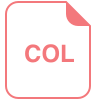
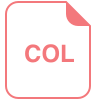