单片机C语言控制语句:if、else、switch和循环,掌握编程流程控制
发布时间: 2024-07-06 22:45:18 阅读量: 56 订阅数: 30 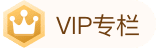
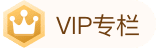
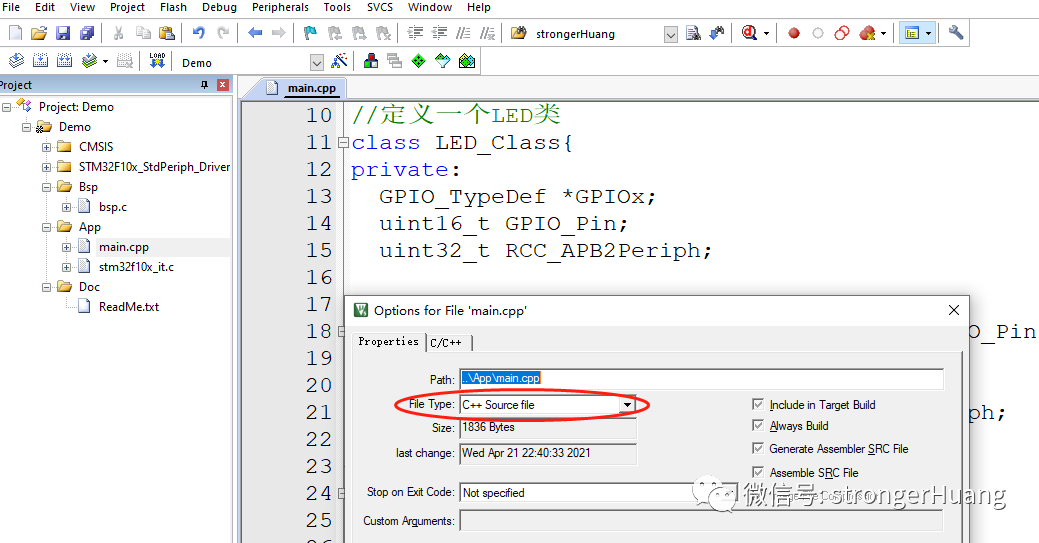
# 1. 单片机C语言控制语句概述**
单片机C语言中的控制语句用于控制程序执行的流程,包括条件控制语句和循环控制语句。条件控制语句根据条件表达式确定执行路径,而循环控制语句重复执行代码块。
控制语句是单片机C语言编程中必不可少的元素,它们使程序能够做出决策、重复任务和处理不同的情况。熟练掌握控制语句对于编写高效、可读且可维护的单片机C语言程序至关重要。
# 2. 单片机C语言条件控制语句
条件控制语句是单片机C语言中用于根据特定条件执行不同代码块的语句。它允许程序根据输入或状态做出决策,从而实现更复杂和动态的行为。
### 2.1 if-else语句
if-else语句是最基本的条件控制语句,它允许程序根据一个条件执行不同的代码块。
#### 2.1.1 if语句的语法和使用
if语句的语法如下:
```c
if (条件) {
// 条件为真的代码块
}
```
其中,`条件`是一个布尔表达式,如果为真,则执行`条件`后的代码块。
**示例:**
```c
int x = 5;
if (x > 0) {
printf("x是正数\n");
}
```
#### 2.1.2 else语句的语法和使用
else语句用于在`if`语句的条件为假时执行另一段代码块。它的语法如下:
```c
if (条件) {
// 条件为真的代码块
} else {
// 条件为假的代码块
}
```
**示例:**
```c
int x = -5;
if (x > 0) {
printf("x是正数\n");
} else {
printf("x是负数\n");
}
```
#### 2.1.3 if-else语句的嵌套使用
if-else语句可以嵌套使用,以处理更复杂的条件。嵌套if-else语句的语法如下:
```c
if (条件1) {
// 条件1为真的代码块
} else if (条件2) {
// 条件2为真的代码块
} else {
// 条件1和条件2都为假的代码块
}
```
**示例:**
```c
int x = 0;
if (x > 0) {
printf("x是正数\n");
} else if (x < 0) {
printf("x是负数\n");
} else {
printf("x是零\n");
}
```
### 2.2 switch语句
switch语句是一种多路分支条件控制语句,它允许程序根据一个变量的值执行不同的代码块。
#### 2.2.1 switch语句的语法和使用
switch语句的语法如下:
```c
switch (变量) {
case 值1:
// 值1匹配时的代码块
break;
case 值2:
// 值2匹配时的代码块
break;
...
default:
// 没有匹配任何值时的代码块
break;
}
```
其中,`变量`是要进行比较的变量,`值1`、`值2`等是与`变量`进行比较的值。
**示例:**
```c
int x = 1;
switch (x) {
case 1:
printf("x是1\n");
break;
case 2:
printf("x是2\n");
break;
default:
printf("x不是1或2\n");
break;
}
```
#### 2.2.2 case语句的语法和使用
case语句用于指定要与`变量`进行比较的值。它的语法如下:
```c
case 值:
// 值匹配时的代码块
break;
```
**示例:**
```c
int x = 1;
switch (x) {
case 1:
printf("x是1\n");
break;
case 2:
printf("x是2\n");
break;
default:
printf("x不是1或2\n");
break;
}
```
#### 2.2.3 default语句的语法和使用
default语句用于指定在`变量`没有匹配任何`case`值时执行的代码块。它的语法如下:
```c
default:
// 没有匹配任何值时的代码块
break;
```
**示例:**
```c
int x = 1;
switch (x) {
case 1:
printf("x是1\n");
break;
case 2:
printf("x是2\n");
break;
default:
printf("x不是1或2\n");
break;
}
```
# 3.1 for循环语句
#### 3.1.1 for循环语句的语法和使用
for循环语句是一种初始化、条件判断和更新操作依次执行的循环控制语句。其语法格式如下:
```c
for (初始化语句; 条件判断语句; 更新语句) {
循环体语句;
}
```
其中:
- 初始化语句:在循环开始前执行一次,通常用于初始化循环变量。
- 条件判断语句:在每次循环开始前执行,如果条件为真,则执行循环体语句,否则退出循环。
- 更新语句:在每次循环体语句执行完成后执行,通常用于更新循环变量。
**示例:**
```c
for (int i = 0; i < 10; i++) {
printf("当前索引值:%d\n", i);
}
```
该代码片段将输出从0到9的索引值。
#### 3.1.2 for循环语句的嵌套使用
for循环语句可以嵌套使用,即在一个for循环体中再嵌套一个或多个for循环。嵌套for循环的执行顺序是从最内层循环开始,依次向外执行。
**示例:**
```c
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 5; j++) {
printf("i = %d, j = %d\n", i, j);
}
}
```
该代码片段将输出如下结果:
```
i = 0, j = 0
i = 0, j = 1
i = 0, j = 2
i = 0, j = 3
i = 0, j = 4
i = 1, j = 0
i = 1, j = 1
i = 1, j = 2
i = 1, j = 3
i = 1, j = 4
i = 2, j = 0
i = 2, j = 1
i = 2, j = 2
i = 2, j = 3
i = 2, j = 4
```
# 4. 单片机C语言控制语句实践应用
### 4.1 条件控制语句的应用
#### 4.1.1 状态机的实现
**状态机**是一种抽象的数学模型,用于描述系统在不同状态之间的转换和行为。在单片机编程中,状态机可以用来实现复杂的控制逻辑。
**使用条件控制语句实现状态机**
```c
enum state {
STATE_INIT,
STATE_IDLE,
STATE_RUNNING,
STATE_FINISHED
};
int main() {
enum state current_state = STATE_INIT;
while (1) {
switch (current_state) {
case STATE_INIT:
// 初始化状态
// ...
current_state = STATE_IDLE;
break;
case STATE_IDLE:
// 空闲状态
// ...
if (条件满足) {
current_state = STATE_RUNNING;
}
break;
case STATE_RUNNING:
// 运行状态
// ...
if (条件满足) {
current_state = STATE_FINISHED;
}
break;
case STATE_FINISHED:
// 结束状态
// ...
current_state = STATE_IDLE;
break;
}
}
return 0;
}
```
**逻辑分析**
该代码使用switch语句实现了一个状态机。程序从STATE_INIT状态开始,然后根据条件切换到不同的状态。每个状态都有自己的行为,并且可以根据条件切换到其他状态。
#### 4.1.2 菜单系统的实现
**菜单系统**允许用户从一系列选项中进行选择。在单片机编程中,菜单系统可以用来提供用户交互界面。
**使用条件控制语句实现菜单系统**
```c
int main() {
int option;
while (1) {
// 显示菜单选项
// ...
// 获取用户输入
// ...
switch (option) {
case 1:
// 选项1
// ...
break;
case 2:
// 选项2
// ...
break;
case 3:
// 选项3
// ...
break;
default:
// 无效选项
// ...
break;
}
}
return 0;
}
```
**逻辑分析**
该代码使用switch语句实现了一个菜单系统。程序显示菜单选项,获取用户输入,然后根据用户输入切换到不同的选项。每个选项都有自己的行为。
### 4.2 循环控制语句的应用
#### 4.2.1 数据的遍历
**数据遍历**是指逐个访问数据集合中的每个元素。在单片机编程中,循环控制语句可以用来遍历数据。
**使用循环控制语句遍历数据**
```c
int array[] = {1, 2, 3, 4, 5};
int main() {
int i;
for (i = 0; i < sizeof(array) / sizeof(int); i++) {
// 访问数组中的每个元素
// ...
}
return 0;
}
```
**逻辑分析**
该代码使用for循环遍历一个数组。循环变量i从0开始,每次循环递增1,直到i等于数组的长度。在每次循环中,程序访问数组中的当前元素。
#### 4.2.2 延时函数的实现
**延时函数**用于在程序中引入一段时间的延迟。在单片机编程中,循环控制语句可以用来实现延时函数。
**使用循环控制语句实现延时函数**
```c
void delay(int ms) {
int i;
for (i = 0; i < ms * 1000; i++) {
// 循环1000次表示延时1毫秒
// ...
}
}
```
**逻辑分析**
该代码使用for循环实现了一个延时函数。循环变量i从0开始,每次循环递增1,直到i等于ms * 1000。在每次循环中,程序执行一个空操作,这将导致程序延迟1毫秒。
# 5. 单片机C语言控制语句进阶应用**
**5.1 控制语句的组合使用**
**5.1.1 条件控制语句和循环控制语句的结合**
条件控制语句和循环控制语句可以组合使用,实现更复杂的控制逻辑。例如,以下代码使用 `for` 循环和 `if` 语句遍历数组,并打印数组中大于 5 的元素:
```c
#include <stdio.h>
int main() {
int arr[] = {1, 3, 5, 7, 9, 11, 13, 15};
int size = sizeof(arr) / sizeof(arr[0]);
for (int i = 0; i < size; i++) {
if (arr[i] > 5) {
printf("%d ", arr[i]);
}
}
return 0;
}
```
**5.1.2 多重条件控制语句的结合**
多个条件控制语句可以结合使用,实现更复杂的条件判断。例如,以下代码使用嵌套的 `if` 语句判断一个数字是否为偶数、奇数或零:
```c
#include <stdio.h>
int main() {
int num;
printf("Enter a number: ");
scanf("%d", &num);
if (num == 0) {
printf("%d is zero.\n", num);
} else if (num % 2 == 0) {
printf("%d is even.\n", num);
} else {
printf("%d is odd.\n", num);
}
return 0;
}
```
**5.2 控制语句的优化**
**5.2.1 控制语句的嵌套优化**
嵌套的控制语句会降低代码的可读性和可维护性。可以通过使用 `switch` 语句或 `goto` 语句来优化嵌套的控制语句。例如,以下代码使用 `switch` 语句优化了嵌套的 `if` 语句:
```c
#include <stdio.h>
int main() {
int num;
printf("Enter a number: ");
scanf("%d", &num);
switch (num) {
case 0:
printf("%d is zero.\n", num);
break;
case 1:
printf("%d is one.\n", num);
break;
case 2:
printf("%d is two.\n", num);
break;
default:
printf("%d is not zero, one, or two.\n", num);
break;
}
return 0;
}
```
**5.2.2 控制语句的代码优化**
控制语句的代码可以通过使用宏、内联函数或汇编代码来优化。例如,以下代码使用宏优化了 `if` 语句:
```c
#include <stdio.h>
#define IF(condition) if (condition)
int main() {
int num;
printf("Enter a number: ");
scanf("%d", &num);
IF(num == 0) {
printf("%d is zero.\n", num);
} else IF(num % 2 == 0) {
printf("%d is even.\n", num);
} else {
printf("%d is odd.\n", num);
}
return 0;
}
```
0
0
相关推荐
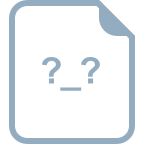
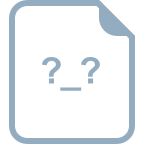
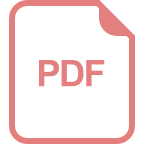





