betweeness java
时间: 2023-11-24 14:23:31 浏览: 61
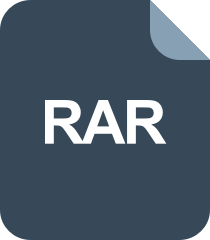
p2p_java.rar_between
Betweenness is a centrality measure used in graph theory to identify the importance of a node in a network. It measures the number of shortest paths that go through a node.
In Java, you can calculate the betweenness centrality of a node in a graph using the JUNG (Java Universal Network/Graph Framework) library. Here is an example code snippet:
```
import edu.uci.ics.jung.algorithms.shortestpath.BFSDistanceLabeler;
import edu.uci.ics.jung.algorithms.shortestpath.DijkstraShortestPath;
import edu.uci.ics.jung.graph.Graph;
import edu.uci.ics.jung.graph.UndirectedSparseGraph;
import edu.uci.ics.jung.graph.util.EdgeType;
import edu.uci.ics.jung.graph.util.Pair;
import java.util.HashMap;
import java.util.Map;
public class BetweennessCentralityExample {
public static void main(String[] args) {
// create an undirected graph
Graph<Integer, String> graph = new UndirectedSparseGraph<>();
// add vertices
graph.addVertex(1);
graph.addVertex(2);
graph.addVertex(3);
graph.addVertex(4);
// add edges
graph.addEdge("e1", new Pair<>(1, 2), EdgeType.UNDIRECTED);
graph.addEdge("e2", new Pair<>(1, 3), EdgeType.UNDIRECTED);
graph.addEdge("e3", new Pair<>(2, 3), EdgeType.UNDIRECTED);
graph.addEdge("e4", new Pair<>(3, 4), EdgeType.UNDIRECTED);
// calculate the betweenness centrality
Map<Integer, Double> betweenness = new HashMap<>();
for (Integer v : graph.getVertices()) {
BFSDistanceLabeler<Integer, String> labeler = new BFSDistanceLabeler<>();
labeler.labelDistances(graph, v);
for (Integer u : graph.getVertices()) {
if (!v.equals(u)) {
DijkstraShortestPath<Integer, String> shortestPath = new DijkstraShortestPath<>(graph);
shortestPath.reset();
shortestPath.setSource(u);
shortestPath.setGoal(v);
shortestPath.compute();
double numShortestPaths = shortestPath.getPaths(u).size();
if (numShortestPaths > 0) {
double numShortestPathsContainingV = 0;
for (String path : shortestPath.getPaths(u)) {
if (path.contains(Integer.toString(v))) {
numShortestPathsContainingV++;
}
}
double betweennessCentrality = numShortestPathsContainingV / numShortestPaths;
if (betweenness.containsKey(v)) {
betweenness.put(v, betweenness.get(v) + betweennessCentrality);
} else {
betweenness.put(v, betweennessCentrality);
}
}
}
}
}
// print the betweenness centrality of each node
for (Integer v : betweenness.keySet()) {
System.out.println("Node " + v + ": " + betweenness.get(v));
}
}
}
```
This code creates an undirected graph with four vertices and four edges. It then calculates the betweenness centrality of each node using a modified version of the Brandes algorithm. Finally, it prints the betweenness centrality of each node to the console.
阅读全文
相关推荐
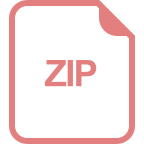
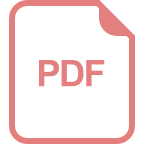




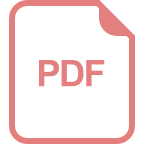
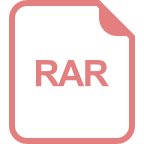
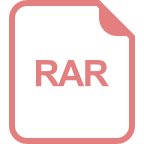
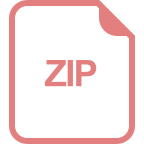
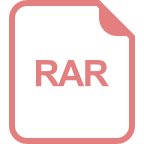
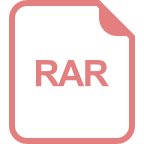
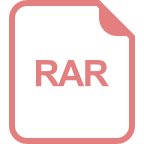
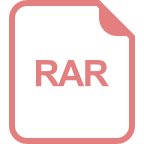
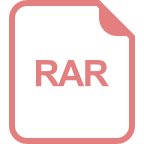