C++定义一个时钟类 Clock ,设计成员函数 SetAlarm ( int hour , int minute , int second ) 设置响铃时间:用 run ()成员函数摸拟时钟运行,当运行到响铃时提示响铃。
时间: 2024-01-29 16:04:20 浏览: 41
以下是一个简单的时钟类 Clock 的实现,包括 SetAlarm 和 run 成员函数:
```cpp
#include <iostream>
#include <ctime>
using namespace std;
class Clock {
private:
int hour;
int minute;
int second;
int alarm_hour;
int alarm_minute;
int alarm_second;
public:
Clock(int h, int m, int s) { // 构造函数,设置当前时间
hour = h;
minute = m;
second = s;
alarm_hour = -1; // 默认不设置闹钟
alarm_minute = -1;
alarm_second = -1;
}
void SetAlarm(int h, int m, int s) { // 设置闹钟
alarm_hour = h;
alarm_minute = m;
alarm_second = s;
}
void run() { // 时钟运行
while (true) {
// 获取当前时间
time_t now = time(nullptr);
tm* ltm = localtime(&now);
int current_hour = ltm->tm_hour;
int current_minute = ltm->tm_min;
int current_second = ltm->tm_sec;
// 显示当前时间
cout << "Current time: " << current_hour << ":" << current_minute << ":" << current_second << endl;
// 判断是否到达设置的闹钟时间
if (current_hour == alarm_hour && current_minute == alarm_minute && current_second == alarm_second) {
cout << "Ring ring ring!" << endl;
break;
}
// 等待一秒钟
sleep(1);
}
}
};
int main() {
// 创建时钟对象,设置当前时间为 10:30:00
Clock clock(10, 30, 0);
// 设置响铃时间为 10:31:00
clock.SetAlarm(10, 31, 0);
// 运行时钟,直到响铃时间到达
clock.run();
return 0;
}
```
在上述代码中,我们使用 time 函数获取当前时间,并使用 sleep 函数等待一秒钟。注意,sleep 函数在 Linux 系统下可用,如果你是在 Windows 下编译运行代码,需要使用 Windows API 的 Sleep 函数代替。
相关推荐
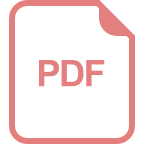














