java 定时抽取一张表的数据插入到另一张表实现
时间: 2023-09-20 22:05:53 浏览: 105
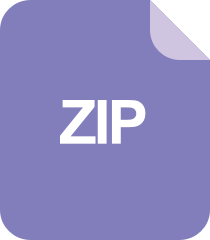
JAVA将一个数据中数据定时自动复制(抽取)到另一个数据库

可以使用 Java 的 Timer 和 TimerTask 类来实现定时任务。
首先,你需要创建一个 Timer 对象,并使用它调度一个 TimerTask 对象,以便在指定的时间间隔内执行任务。在 TimerTask 中,你可以编写代码来抽取一张表的数据并插入到另一张表中。
以下是一个示例代码,可以每隔一分钟从表A中抽取数据并插入到表B中:
```java
import java.sql.*;
public class TableDataTransfer {
private static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
private static final String DB_URL = "jdbc:mysql://localhost:3306/";
private static final String USER = "root";
private static final String PASS = "password";
private static final String SOURCE_TABLE = "table_a";
private static final String DEST_TABLE = "table_b";
public static void main(String[] args) {
Timer timer = new Timer();
TimerTask task = new TimerTask() {
@Override
public void run() {
Connection conn = null;
Statement stmt = null;
try {
// Register JDBC driver
Class.forName(JDBC_DRIVER);
// Open a connection
System.out.println("Connecting to database...");
conn = DriverManager.getConnection(DB_URL, USER, PASS);
// Execute a query to get data from source table
System.out.println("Extracting data from source table...");
stmt = conn.createStatement();
String sql = "SELECT * FROM " + SOURCE_TABLE;
ResultSet rs = stmt.executeQuery(sql);
// Insert data into destination table
System.out.println("Inserting data into destination table...");
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
String insertSql = "INSERT INTO " + DEST_TABLE + " (id, name, age) VALUES (" + id + ", '" + name + "', " + age + ")";
stmt.executeUpdate(insertSql);
}
// Clean-up environment
rs.close();
stmt.close();
conn.close();
} catch (SQLException se) {
// Handle errors for JDBC
se.printStackTrace();
} catch (Exception e) {
// Handle errors for Class.forName
e.printStackTrace();
} finally {
// Finally block used to close resources
try {
if (stmt != null)
stmt.close();
} catch (SQLException se2) {
} // nothing we can do
try {
if (conn != null)
conn.close();
} catch (SQLException se) {
se.printStackTrace();
} // end finally try
}
}
};
// Schedule the task to run every minute
timer.schedule(task, 0, 60 * 1000);
}
}
```
请注意,这只是一个基本示例代码,你需要根据实际情况进行修改和优化。例如,你可以添加异常处理、日志记录等功能来提高代码的健壮性。
阅读全文
相关推荐
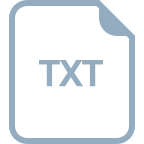


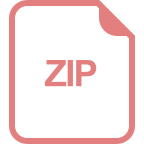
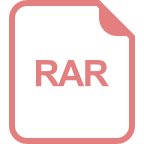
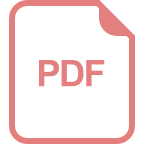
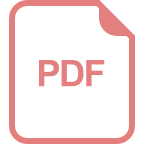
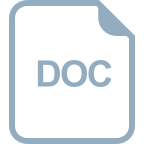
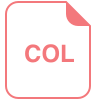
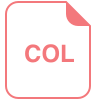
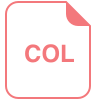
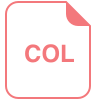
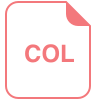
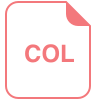
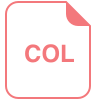
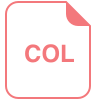
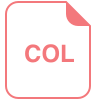
