幫我寫用pytorch,vgg16,gpu訓練,實現two stream early fusion,我發現之前跑得時候出現rgb圖像channel數與光流圖像channel不同而造成無法融合,有建議方法?
时间: 2023-11-06 13:08:25 浏览: 42
首先,确保你的RGB图像和光流图像具有相同数量的通道,例如都是3通道。如果不是,则需要通过转换来使它们具有相同数量的通道。
其次,实现Two-Stream Early Fusion,可以按照以下步骤进行:
1. 定义两个分支网络:一个处理RGB图像,另一个处理光流图像。在这里,我们使用VGG16作为我们的分支网络。
2. 对于每个分支网络,将其前几层冻结,并添加一个全局平均池化层和一个全连接层。这些层将作为特征提取器,以提取RGB图像和光流图像的特征。
3. 将两个分支网络的输出连接起来,并添加一个全连接层和一个softmax层,以进行分类。
4. 定义您的优化器和损失函数。在这里,我们使用交叉熵损失函数和Adam优化器。
5. 在GPU上训练模型。在PyTorch中,您可以使用.cuda()方法将模型和数据移动到GPU上。
下面是一个简单的代码示例,可以帮助您开始:
```python
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision.models import vgg16
# Define the Two-Stream Early Fusion model
class TwoStreamEarlyFusion(nn.Module):
def __init__(self, num_classes):
super(TwoStreamEarlyFusion, self).__init__()
# Define the RGB branch
self.rgb = vgg16(pretrained=True)
self.rgb.classifier = nn.Sequential(
nn.Dropout(),
nn.Linear(25088, 4096),
nn.ReLU(inplace=True),
nn.Dropout(),
nn.Linear(4096, 4096),
nn.ReLU(inplace=True),
nn.Linear(4096, num_classes),
)
# Define the optical flow branch
self.flow = vgg16(pretrained=True)
self.flow.classifier = nn.Sequential(
nn.Dropout(),
nn.Linear(25088, 4096),
nn.ReLU(inplace=True),
nn.Dropout(),
nn.Linear(4096, 4096),
nn.ReLU(inplace=True),
nn.Linear(4096, num_classes),
)
# Define the fusion layer
self.fusion = nn.Sequential(
nn.Linear(num_classes*2, num_classes),
nn.Softmax(dim=1)
)
def forward(self, rgb, flow):
rgb_feat = self.rgb.features(rgb)
rgb_feat = self.rgb.avgpool(rgb_feat)
rgb_feat = torch.flatten(rgb_feat, 1)
rgb_feat = self.rgb.classifier(rgb_feat)
flow_feat = self.flow.features(flow)
flow_feat = self.flow.avgpool(flow_feat)
flow_feat = torch.flatten(flow_feat, 1)
flow_feat = self.flow.classifier(flow_feat)
fusion_feat = torch.cat((rgb_feat, flow_feat), dim=1)
fusion_feat = self.fusion(fusion_feat)
return fusion_feat
# Move the model and data to GPU
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
model = TwoStreamEarlyFusion(num_classes=10)
model.to(device)
# Define the optimizer and loss function
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
# Train the model
for epoch in range(num_epochs):
running_loss = 0.0
for i, data in enumerate(trainloader, 0):
# Get the inputs
rgb, flow, labels = data
rgb, flow, labels = rgb.to(device), flow.to(device), labels.to(device)
# Zero the parameter gradients
optimizer.zero_grad()
# Forward + backward + optimize
outputs = model(rgb, flow)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
# Print statistics
running_loss += loss.item()
if i % 100 == 99: # Print every 100 mini-batches
print('[%d, %5d] loss: %.3f' %
(epoch + 1, i + 1, running_loss / 100))
running_loss = 0.0
print('Finished Training')
```
相关推荐
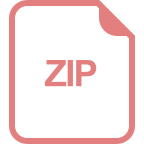














