基于scrapy开发一个微信公众号爬虫程序
时间: 2024-06-08 17:05:49 浏览: 27
好的,下面是一个基于Scrapy框架开发的微信公众号爬虫程序的示例代码:
首先,我们需要在命令行中安装Scrapy和其他相关依赖:
```
pip install scrapy
pip install scrapy-user-agents
pip install scrapy-redis
pip install scrapy-splash
```
然后,创建一个新的Scrapy项目:
```
scrapy startproject wechat_spider
```
接下来,进入项目目录并创建一个Spider:
```
cd wechat_spider
scrapy genspider wechat_spider weixin.sogou.com
```
在Spider中,我们需要定义要爬取的URL和页面元素的解析方法。下面是示例代码:
```python
import scrapy
from scrapy_splash import SplashRequest
from scrapy_redis.spiders import RedisSpider
class WechatSpider(RedisSpider):
name = 'wechat_spider'
allowed_domains = ['weixin.sogou.com']
start_urls = ['https://weixin.sogou.com/']
def parse(self, response):
# 构造搜狗微信搜索的URL
keyword = 'Python'
url = f'https://weixin.sogou.com/weixin?type=1&s_from=input&query={keyword}&ie=utf8&_sug_=n&_sug_type_='
yield SplashRequest(url, self.parse_search_result, args={'wait': 10})
def parse_search_result(self, response):
# 解析搜狗微信搜索结果页面
articles = response.css('.news-box .news-list li .txt-box h3 a::attr(href)').getall()
for article_url in articles:
yield SplashRequest(article_url, self.parse_article, args={'wait': 10})
def parse_article(self, response):
# 解析公众号文章页面
title = response.css('#activity-name::text').get()
content = response.css('#js_content').get()
yield {'title': title, 'content': content}
```
在这个示例中,我们使用了Scrapy-Redis、Scrapy-Splash和Scrapy-User-Agents等扩展库。我们首先在parse方法中构造搜狗微信搜索的URL,并使用SplashRequest发送请求。在parse_search_result方法中,我们解析搜狗微信搜索结果页面,获取每篇文章的URL,并再次使用SplashRequest发送请求。最后,在parse_article方法中,我们解析公众号文章页面,获取文章标题和内容,并通过yield返回给Scrapy框架。
在运行爬虫之前,我们需要在settings.py中配置Redis和Splash的相关参数:
```python
# Redis配置
REDIS_HOST = 'localhost'
REDIS_PORT = 6379
REDIS_PARAMS = {'password': 'your_password'}
# Splash配置
SPLASH_URL = 'http://localhost:8050'
# 下载中间件配置
DOWNLOADER_MIDDLEWARES = {
'scrapy_user_agents.middlewares.RandomUserAgentMiddleware': 400,
'scrapy_splash.SplashCookiesMiddleware': 723,
'scrapy_splash.SplashMiddleware': 725,
'scrapy.downloadermiddlewares.httpcompression.HttpCompressionMiddleware': 810,
}
# 爬虫中间件配置
SPIDER_MIDDLEWARES = {
'scrapy_splash.SplashDeduplicateArgsMiddleware': 100,
}
# Splash参数配置
SPLASH_ARGS = {
'wait': 5,
'images': 0,
'render_all': 1,
'lua_source': """
function main(splash, args)
splash.private_mode_enabled = false
assert(splash:go(args.url))
assert(splash:wait(args.wait))
return splash:html()
end
""",
}
```
最后,使用以下命令启动爬虫:
```
scrapy crawl wechat_spider
```
这是一个简单的微信公众号爬虫程序示例,你可以根据自己的需求进行修改和扩展。
相关推荐
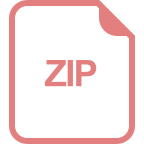









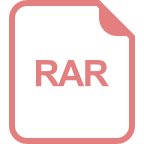
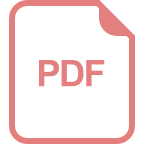
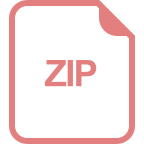
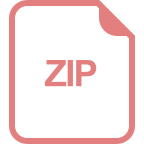