#include <iostream> using namespace std; class MyClass { int* p; const int N; public: // ERROR **********found********** MyClass(int val) : N=1 { p = new int; *p = val; } // ERROR **********found********** ~MyClass() { delete *p; } friend void print(MyClass& obj); }; // ERROR **********found********** void MyClass::print(MyClass& obj) { cout << "value = " << *(obj.p) << endl; cout << "number = " << obj.N << endl; } int main() { MyClass obj(63); print(obj); return 0; }
时间: 2023-06-29 22:16:18 浏览: 52
在这段代码中,有三个错误:
1. 在 MyClass 类的构造函数中,使用了 const 成员初始化列表,但是 N 是一个非静态成员变量,不能在初始化列表中初始化。正确的做法是在构造函数的函数体中给 N 赋值。
2. 在 MyClass 类的析构函数中,使用了 delete *p,但是 p 是一个指向 int 的指针,应该使用 delete p。
3. 在 MyClass 类的友元函数 print() 中,应该将其定义为 MyClass 类的成员函数,而不是独立的函数。
下面是修改后的代码:
```cpp
#include <iostream>
using namespace std;
class MyClass {
int* p;
const int N;
public:
MyClass(int val) : N(1) {
p = new int;
*p = val;
}
~MyClass() { delete p; }
void print() {
cout << "value = " << *p << endl;
cout << "number = " << N << endl;
}
};
int main() {
MyClass obj(63);
obj.print();
return 0;
}
```
相关问题
#include <iostream> using namespace std; /********* Begin *********/ class Complex
#include <iostream> 是C++语言中的一个预处理指令,用于引入标准输入输出流库。其中,iostream头文件定义了cin、cout、cerr和clog对象,分别代表标准输入流、标准输出流、非缓存错误输出流和缓存错误输出流。使用这些对象可以进行输入输出操作。
现在来介绍一下class Complex,它是一个复数类,可以表示实部和虚部为双精度数的复数。在这个类中,定义了一些成员函数,如重载运算符、计算模长、输出复数等。
注:如果需要了解更详细的内容,可以阅读C++相关教材或者参考CSDN社区中其他开发者的相关文章。
#include <iostream> using namespace std; int main() {
这是一个C++程序,它的功能是输出一个n*n的矩阵,其中每个元素都是从n*n开始递减1的数字。下面是代码的解释:
```c++
#include <iostream> // 引入iostream库,用于输入输出
#include <iomanip> // 引入iomanip库,用于格式化输出
using namespace std; // 使用std命名空间
int main() { // 主函数
int n,i,k=0; // 定义整型变量n、、k,并初始化k为0
cin>>n; // 从标准输入中读取n的值
for(i=n*n;i>=1;i--) // 循环n*n次,从n*n开始递减1
{
cout<<setw(5)<<i; // 输出当前数字,setw(5)用于设置输出宽度为5
k++; // k加1
if(k%n==0) // 如果k是n的倍数,说明当前行已经输出完毕
cout<<endl; // 输出换行符
}
cout<<endl; // 输出一个额外的换行符
return 0; // 返回0,表示程序正常结束
}
```
相关推荐
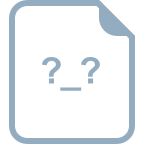
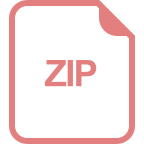











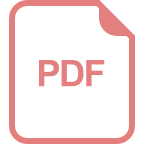