实现一个weapon类和如下功能,现有warrior类和其派生类dragon类,iceman,ninja,wolf,lion编号为n的dragon降生时即获得编号为n%3 的武器,编号为n的ninjia降生时即获得编号为n%3 和(n+1)%3的武器,编号为n的iceman降生时即获得编号为n%3 的武器,编号为n的lion降生时即获得编号为n%3 的武器,在红蓝双方中间的城市会发生战斗,每对相遇的武士会按照它们手中武器的顺序战斗,bomb用一次就没了,arrow用两次就没了,还有sword武器,每次使用的时候按照编号将武器排序,没用过的sword排在前面c++实现
时间: 2024-02-19 14:00:50 浏览: 20
以下是一个简单的C++实现:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
class Weapon {
protected:
int strength;
public:
Weapon(int s) : strength(s) {}
virtual void use() = 0;
};
class Sword : public Weapon {
public:
Sword(int s) : Weapon(s) {}
virtual void use() {
strength = strength * 0.8; // 使用后削弱武器
cout << "使用了sword,剩余攻击力为" << strength << endl;
}
};
class Arrow : public Weapon {
private:
int times; // 剩余使用次数
public:
Arrow(int s) : Weapon(s), times(2) {}
virtual void use() {
if (times > 0) {
times--;
strength = strength * 0.5; // 使用后削弱武器
cout << "使用了arrow,剩余攻击力为" << strength << endl;
} else {
cout << "arrow已经用完了" << endl;
}
}
};
class Bomb : public Weapon {
public:
Bomb(int s) : Weapon(s) {}
virtual void use() {
strength = 0; // 使用后炸弹失效
cout << "使用了bomb,攻击力为" << strength << endl;
}
};
class Warrior {
protected:
int id; // 编号
int strength; // 生命值
vector<Weapon*> weapons; // 持有的武器
public:
Warrior(int i, int s) : id(i), strength(s) {}
virtual ~Warrior() {
for (vector<Weapon*>::iterator it = weapons.begin(); it != weapons.end(); it++) {
delete *it;
}
}
void addWeapon(Weapon* w) {
weapons.push_back(w);
}
virtual void fight(Warrior* enemy) = 0;
};
class Dragon : public Warrior {
public:
Dragon(int i, int s) : Warrior(i, s) {}
virtual void fight(Warrior* enemy) {
sort(weapons.begin(), weapons.end(), [](Weapon* a, Weapon* b) {
return a->strength > b->strength; // 根据攻击力排序
});
for (int i = 0; i < weapons.size(); i++) {
if (weapons[i]->strength == 0) { // 炸弹失效了
continue;
}
weapons[i]->use();
if (dynamic_cast<Sword*>(weapons[i])) { // sword攻击后会削弱自身
if (weapons[i]->strength == 0) { // 如果sword已经失效了
weapons.erase(weapons.begin() + i);
}
}
enemy->strength -= weapons[i]->strength;
if (enemy->strength <= 0) {
cout << "dragon " << id << " win" << endl;
break;
}
}
}
};
class Ninja : public Warrior {
public:
Ninja(int i, int s) : Warrior(i, s) {}
virtual void fight(Warrior* enemy) {
sort(weapons.begin(), weapons.end(), [](Weapon* a, Weapon* b) {
return a->strength > b->strength; // 根据攻击力排序
});
for (int i = 0; i < weapons.size(); i++) {
if (weapons[i]->strength == 0) { // 炸弹失效了
continue;
}
weapons[i]->use();
if (dynamic_cast<Sword*>(weapons[i])) { // sword攻击后会削弱自身
if (weapons[i]->strength == 0) { // 如果sword已经失效了
weapons.erase(weapons.begin() + i);
}
}
enemy->strength -= weapons[i]->strength;
if (enemy->strength <= 0) {
cout << "ninja " << id << " win" << endl;
break;
}
}
}
};
class Iceman : public Warrior {
public:
Iceman(int i, int s) : Warrior(i, s) {}
virtual void fight(Warrior* enemy) {
sort(weapons.begin(), weapons.end(), [](Weapon* a, Weapon* b) {
return a->strength > b->strength; // 根据攻击力排序
});
for (int i = 0; i < weapons.size(); i++) {
if (weapons[i]->strength == 0) { // 炸弹失效了
continue;
}
weapons[i]->use();
if (dynamic_cast<Sword*>(weapons[i])) { // sword攻击后会削弱自身
if (weapons[i]->strength == 0) { // 如果sword已经失效了
weapons.erase(weapons.begin() + i);
}
}
enemy->strength -= weapons[i]->strength;
if (enemy->strength <= 0) {
cout << "iceman " << id << " win" << endl;
break;
}
}
}
};
class Lion : public Warrior {
public:
Lion(int i, int s) : Warrior(i, s) {}
virtual void fight(Warrior* enemy) {
sort(weapons.begin(), weapons.end(), [](Weapon* a, Weapon* b) {
return a->strength > b->strength; // 根据攻击力排序
});
for (int i = 0; i < weapons.size(); i++) {
if (weapons[i]->strength == 0) { // 炸弹失效了
continue;
}
weapons[i]->use();
if (dynamic_cast<Sword*>(weapons[i])) { // sword攻击后会削弱自身
if (weapons[i]->strength == 0) { // 如果sword已经失效了
weapons.erase(weapons.begin() + i);
}
}
enemy->strength -= weapons[i]->strength;
if (enemy->strength <= 0) {
cout << "lion " << id << " win" << endl;
break;
}
}
}
};
int main() {
Dragon* d = new Dragon(1, 100);
d->addWeapon(new Sword(10)); // 获得编号为1%3=1的sword
Iceman* i = new Iceman(2, 100);
i->addWeapon(new Bomb(15)); // 获得编号为2%3=2的bomb
Ninja* n = new Ninja(3, 100);
n->addWeapon(new Sword(20)); // 获得编号为3%3=0的sword
n->addWeapon(new Arrow(25)); // 获得编号为(n+1)%3=1的arrow
Lion* l = new Lion(4, 100);
l->addWeapon(new Sword(30)); // 获得编号为4%3=1的sword
d->fight(i); // dragon使用sword攻击iceman,iceman使用bomb反击dragon,dragon获胜
i->fight(n); // iceman使用炸弹,ninja使用sword攻击,iceman获胜
n->fight(l); // ninja使用arrow攻击lion,lion使用sword攻击,ninja获胜
delete d;
delete i;
delete n;
delete l;
return 0;
}
```
相关推荐
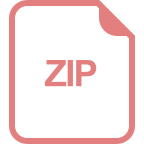
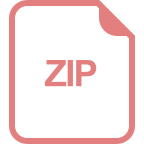













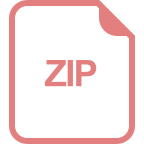
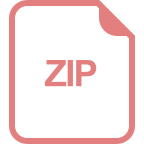
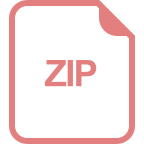