基于RS485通信的Modbus通信协议的C语言例程
时间: 2024-01-23 16:03:51 浏览: 47
以下是一个基于RS485通信的Modbus通信协议的C语言例程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define RS485_DEV "/dev/ttyUSB0" // 串口设备文件路径
#define BAUD_RATE B9600 // 波特率
#define DATA_BITS CS8 // 数据位数
#define STOP_BITS 1 // 停止位数
#define PARITY_NONE 0 // 校验位
int fd; // 串口文件描述符
// 打开串口函数
int open_port(char *port)
{
int fd;
fd = open(port, O_RDWR | O_NOCTTY);
if (fd < 0) {
perror("open_port: Unable to open serial port");
return -1;
}
struct termios options;
tcgetattr(fd, &options);
// 设置波特率
cfsetispeed(&options, BAUD_RATE);
cfsetospeed(&options, BAUD_RATE);
// 设置数据位、停止位和校验位
options.c_cflag &= ~CSIZE;
options.c_cflag |= DATA_BITS;
options.c_cflag &= ~(PARENB | PARODD);
options.c_cflag |= PARITY_NONE;
options.c_cflag &= ~CSTOPB;
// 设置为非规范模式和无缓冲模式
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
options.c_cc[VMIN] = 0;
options.c_cc[VTIME] = 10;
// 设置为原始模式
options.c_oflag &= ~OPOST;
options.c_iflag &= ~(INLCR | ICRNL | IGNCR);
options.c_iflag &= ~(IXON | IXOFF | IXANY);
tcsetattr(fd, TCSANOW, &options);
return fd;
}
// 发送Modbus命令函数
int send_modbus_cmd(unsigned char *cmd, int cmd_len, unsigned char *rx_buf, int rx_len)
{
// 设置RS485为发送模式
ioctl(fd, TIOCMGET, &status);
status |= TIOCM_RTS;
ioctl(fd, TIOCMSET, &status);
// 发送Modbus命令
write(fd, cmd, cmd_len);
// 等待接收Modbus响应
usleep(10000);
// 设置RS485为接收模式
ioctl(fd, TIOCMGET, &status);
status &= ~TIOCM_RTS;
ioctl(fd, TIOCMSET, &status);
// 接收Modbus响应
int rxed = read(fd, rx_buf, rx_len);
return rxed;
}
int main(int argc, char *argv[])
{
unsigned char cmd[8] = {0x01, 0x03, 0x00, 0x00, 0x00, 0x01, 0x84, 0x0A}; // Modbus命令
unsigned char rx_buf[1024]; // 接收缓冲区
fd = open_port(RS485_DEV); // 打开串口
if (fd < 0) {
printf("Failed to open serial port\n");
return -1;
}
int rxed = send_modbus_cmd(cmd, sizeof(cmd), rx_buf, sizeof(rx_buf)); // 发送Modbus命令并接收响应
if (rxed < 0) {
printf("Failed to send Modbus command\n");
return -1;
}
printf("Modbus response: ");
for (int i = 0; i < rxed; i++) {
printf("%02X ", rx_buf[i]);
}
printf("\n");
close(fd); // 关闭串口
return 0;
}
```
以上例程实现了发送一个读取线圈状态的Modbus命令并接收响应。其中,`open_port`函数用于打开串口并设置其通信参数,`send_modbus_cmd`函数用于发送Modbus命令并接收响应。在发送Modbus命令前,需要将RS485设置为发送模式,接收响应后需要将其设置为接收模式。
相关推荐
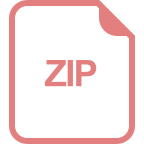














