C语言设是平面上散列的个点的集合。请编写程序找出集合中距离最近的点对的距离。 输入格式: 输入第一行给出一个正整数,表示平面上点的数量。随后行,每行给出一个实数对,每个实数对表示一个点的坐标值,其中第1数表示横坐标,第2数表示纵坐标。 输出格式: 最近点对中两个点之间的距离。
时间: 2024-03-18 11:38:29 浏览: 58
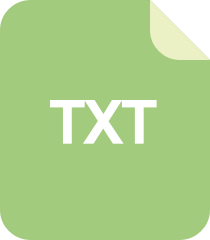
C语言:计算空间两点之间的距离
以下是一个基于分治算法实现的 C 语言程序,可以解决这个问题:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
// 定义一个结构体表示平面上的一个点
typedef struct {
double x;
double y;
} Point;
// 按照 x 坐标排序
int cmp_x(const void* a, const void* b) {
Point* p1 = (Point*) a;
Point* p2 = (Point*) b;
if (p1->x < p2->x) return -1;
else if (p1->x > p2->x) return 1;
else return 0;
}
// 按照 y 坐标排序
int cmp_y(const void* a, const void* b) {
Point* p1 = (Point*) a;
Point* p2 = (Point*) b;
if (p1->y < p2->y) return -1;
else if (p1->y > p2->y) return 1;
else return 0;
}
// 计算两点之间的距离
double dist(Point* p1, Point* p2) {
double dx = p1->x - p2->x;
double dy = p1->y - p2->y;
return sqrt(dx*dx + dy*dy);
}
// 分治算法求解最近点对问题
double closest_pair(Point* p, int n) {
if (n <= 1) return INFINITY;
int mid = n / 2;
double midx = p[mid].x;
double d = fmin(closest_pair(p, mid), closest_pair(p+mid, n-mid));
qsort(p, n, sizeof(Point), cmp_y);
Point* strip = (Point*) malloc(sizeof(Point) * n);
int j = 0;
for (int i = 0; i < n; i++) {
if (fabs(p[i].x - midx) < d) {
for (int k = j-1; k >= 0 && p[i].y - strip[k].y < d; k--)
d = fmin(d, dist(&p[i], &strip[k]));
strip[j++] = p[i];
}
}
free(strip);
return d;
}
int main() {
int n;
scanf("%d", &n);
Point* p = (Point*) malloc(sizeof(Point) * n);
for (int i = 0; i < n; i++)
scanf("%lf %lf", &p[i].x, &p[i].y);
qsort(p, n, sizeof(Point), cmp_x);
double d = closest_pair(p, n);
printf("%.2lf\n", d);
free(p);
return 0;
}
```
该程序的时间复杂度为 $O(n\log n)$,其中 $n$ 是平面上点的数量。
阅读全文
相关推荐
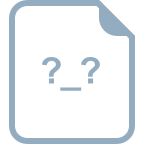
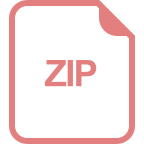















