用java写一个Prim算法
时间: 2023-09-22 13:06:27 浏览: 147
### 回答1:
对于Prim算法,你可以使用Java中的图论算法包(jgrapht)来实现。它可以帮助你实现Prim算法,以最小生成树的形式构建图。你可以参考以下代码:
import org.jgrapht.alg.interfaces.MinimumSpanningTree;
import org.jgrapht.alg.spanning.PrimMinimumSpanningTree;
import org.jgrapht.graph.DefaultWeightedEdge;
import org.jgrapht.graph.SimpleWeightedGraph;
//Create a simple weighted graph
SimpleWeightedGraph<String, DefaultWeightedEdge> graph = new SimpleWeightedGraph<>(DefaultWeightedEdge.class);
//Add vertices and edges to the graph
//...
//Create a Prim's algorithm object
MinimumSpanningTree<String, DefaultWeightedEdge> prim = new PrimMinimumSpanningTree<>(graph);
//Compute the minimum spanning tree
Set<DefaultWeightedEdge> mst = prim.getMinimumSpanningTreeEdgeSet();
### 回答2:
Prim算法是一种用于解决最小生成树问题的算法,它的目标是找出一个无向图的最小连接子图,同时保证子图中的所有节点联通且总权值最小。
下面是使用Java语言实现Prim算法的基本步骤:
1. 创建一个ArrayList集合,用于存储最小生成树的边。
2. 创建一个Boolean型的数组visited,用于标记节点是否被访问过。
3. 创建一个优先队列,用于存储待选边。
4. 将起始节点标记为已访问,并将其所有边加入优先队列。
5. 不断循环以下操作直到优先队列为空:
- 从优先队列中取出权值最小的边。
- 若该边的另一端节点未被访问过,则将其标记为已访问,并将该边加入最小生成树的边集合中。
- 将该边的另一端节点的所有边加入优先队列。
6. 返回最小生成树的边集合。
下面是一个简单的Java代码实现:
```java
import java.util.ArrayList;
import java.util.PriorityQueue;
class Edge implements Comparable<Edge> {
int from;
int to;
int weight;
public Edge(int from, int to, int weight) {
this.from = from;
this.to = to;
this.weight = weight;
}
public int compareTo(Edge o) {
return this.weight - o.weight;
}
}
public class PrimAlgorithm {
public static ArrayList<Edge> prim(int[][] graph) {
int n = graph.length;
ArrayList<Edge> mst = new ArrayList<>();
boolean[] visited = new boolean[n];
PriorityQueue<Edge> pq = new PriorityQueue<>();
visited[0] = true;
for (int i = 0; i < n; i++) {
if (graph[0][i] != 0) {
pq.add(new Edge(0, i, graph[0][i]));
}
}
while (!pq.isEmpty()) {
Edge edge = pq.poll();
int nextNode = edge.to;
if (!visited[nextNode]) {
visited[nextNode] = true;
mst.add(edge);
for (int i = 0; i < n; i++) {
if (graph[nextNode][i] != 0) {
pq.add(new Edge(nextNode, i, graph[nextNode][i]));
}
}
}
}
return mst;
}
public static void main(String[] args) {
int[][] graph = {
{0, 2, 3, 0},
{2, 0, 0, 1},
{3, 0, 0, 4},
{0, 1, 4, 0}
};
ArrayList<Edge> mst = prim(graph);
for (Edge edge : mst) {
System.out.println(edge.from + " - " + edge.to + ": " + edge.weight);
}
}
}
```
以上是一个简单的Prim算法的Java实现,可以根据需求进行修改和扩展。
### 回答3:
Prim算法是一种常用于解决最小生成树问题的算法。下面是一个用Java编写的Prim算法的示例:
```java
import java.util.ArrayList;
import java.util.PriorityQueue;
class Edge {
int v;
int weight;
public Edge(int v, int weight) {
this.v = v;
this.weight = weight;
}
}
public class PrimAlgorithm {
public static void primMST(int[][] graph) {
int V = graph.length;
boolean[] visited = new boolean[V];
int[] parent = new int[V];
int[] key = new int[V];
for (int i = 0; i < V; i++) {
key[i] = Integer.MAX_VALUE;
visited[i] = false;
}
key[0] = 0;
parent[0] = -1;
PriorityQueue<Edge> pq = new PriorityQueue<>((a, b) -> a.weight - b.weight);
pq.add(new Edge(0, 0));
while (!pq.isEmpty()) {
int u = pq.poll().v;
visited[u] = true;
for (int v = 0; v < V; v++) {
if (graph[u][v] != 0 && !visited[v] && graph[u][v] < key[v]) {
parent[v] = u;
key[v] = graph[u][v];
pq.add(new Edge(v, key[v]));
}
}
}
// 打印最小生成树
System.out.println("边\t权重");
for (int i = 1; i < V; i++) {
System.out.println(parent[i] + " - " + i + "\t" + graph[i][parent[i]]);
}
}
public static void main(String[] args) {
int[][] graph = {
{0, 2, 0, 6, 0},
{2, 0, 3, 8, 5},
{0, 3, 0, 0, 7},
{6, 8, 0, 0, 9},
{0, 5, 7, 9, 0}
};
primMST(graph);
}
}
```
在这个示例中,我们定义了一个`Edge`类来保存边的信息。然后,在`primMST`方法中,我们首先初始化一些数组来跟踪访问的顶点,父节点以及权重;然后,我们使用`PriorityQueue`来保存顶点并根据权重排序。接下来,我们从队列中选取权重最小的顶点并将其标记为已访问,然后遍历与该顶点相连接的顶点。如果发现连接的顶点尚未被访问且权重小于其父节点权重,则更新相应的数组。最后,我们打印出最小生成树的边及其权重。
在`main`方法中,我们创建了一个图示例,并调用`primMST`方法来执行Prim算法,输出最小生成树的边及其权重。
阅读全文
相关推荐
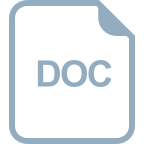
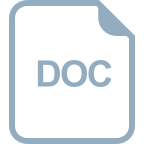
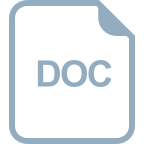


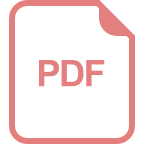
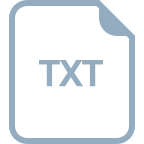
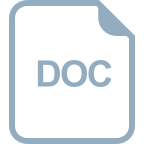
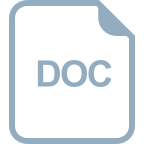







