bmp图像旋转90度c++
时间: 2023-11-03 08:10:27 浏览: 180
bmp图像旋转90度可以通过以下步骤来实现:
1. 读取bmp图像的头文件,获取图像的宽度、高度、像素位数等信息;
2. 创建一个新的bmp图像文件,将宽度和高度交换,像素位数保持不变;
3. 逐行读取原始bmp图像中的像素数据,并将其逆时针旋转90度后写入新的bmp图像中;
4. 保存新的bmp图像文件。
以下是一个简单的C++代码示例,可以实现bmp图像逆时针旋转90度:
```c++
#include <iostream>
#include <fstream>
#include <vector>
using namespace std;
#pragma pack(push, 1)
// 定义bmp文件头结构体
struct BMPFileHeader {
char type[2];
uint32_t size;
uint16_t reserved1;
uint16_t reserved2;
uint32_t offbits;
};
// 定义bmp信息头结构体
struct BMPInfoHeader {
uint32_t size;
int32_t width;
int32_t height;
uint16_t planes;
uint16_t bit_count;
uint32_t compression;
uint32_t size_image;
int32_t x_pels_per_meter;
int32_t y_pels_per_meter;
uint32_t clr_used;
uint32_t clr_important;
};
// 定义RGB颜色结构体
struct RGBColor {
uint8_t b;
uint8_t g;
uint8_t r;
};
#pragma pack(pop)
// 将bmp图像逆时针旋转90度
void rotateImage(const char* input_filename, const char* output_filename) {
// 打开原始bmp图像文件
ifstream input_file(input_filename, ios::binary);
if (!input_file) {
cerr << "Failed to open input file!" << endl;
return;
}
// 读取bmp文件头信息
BMPFileHeader file_header;
input_file.read(reinterpret_cast<char*>(&file_header), sizeof(BMPFileHeader));
// 读取bmp信息头信息
BMPInfoHeader info_header;
input_file.read(reinterpret_cast<char*>(&info_header), sizeof(BMPInfoHeader));
// 获取原始bmp图像的宽度和高度
int32_t width = info_header.width;
int32_t height = info_header.height;
// 创建新的bmp图像文件
ofstream output_file(output_filename, ios::binary);
if (!output_file) {
cerr << "Failed to create output file!" << endl;
return;
}
// 将宽度和高度互换,像素位数不变
info_header.width = height;
info_header.height = width;
// 写入新的bmp文件头信息
output_file.write(reinterpret_cast<const char*>(&file_header), sizeof(BMPFileHeader));
output_file.write(reinterpret_cast<const char*>(&info_header), sizeof(BMPInfoHeader));
// 计算每行像素数据的字节数
int row_size = (width * 3 + 3) & (~3);
// 分配缓冲区用于存储一行像素数据
vector<RGBColor> row_data(row_size / 3);
// 逐行读取原始bmp图像中的像素数据,并将其逆时针旋转90度后写入新的bmp图像中
for (int y = 0; y < height; ++y) {
// 读取一行像素数据
input_file.read(reinterpret_cast<char*>(row_data.data()), row_size);
// 逐列将像素数据写入新的bmp图像中
for (int x = 0; x < width; ++x) {
RGBColor color = row_data[x];
output_file.write(reinterpret_cast<const char*>(&color), sizeof(RGBColor));
}
}
// 关闭文件
input_file.close();
output_file.close();
}
int main() {
rotateImage("input.bmp", "output.bmp");
return 0;
}
```
需要注意的是,该示例代码只适用于24位真彩色的bmp图像。如果bmp图像的像素位数不同,需要对代码进行相应的修改。
阅读全文
相关推荐
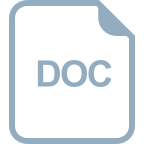
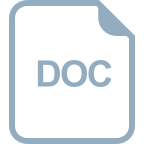
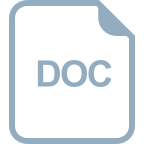


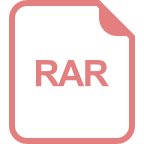
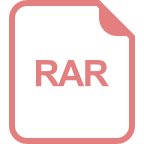

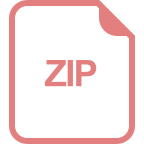
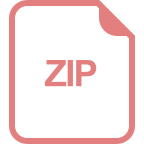
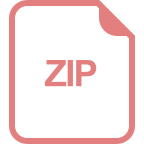
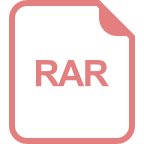
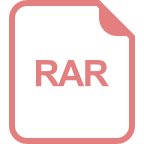
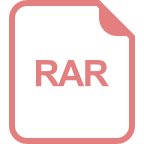
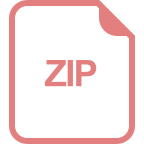
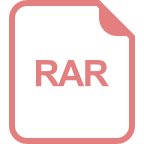
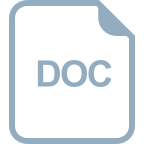
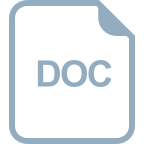