给这段代码添加用户管理
时间: 2023-12-26 15:21:11 浏览: 37
好的,为了添加用户管理,我们需要在代码中添加一个新的结构体来表示用户,并在主函数中添加一些新的选项,例如添加用户、显示用户、删除用户等等。
下面是修改后的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100 // 最大书籍数量
#define MAX_USERS 50 // 最大用户数量
struct Book {
int id;
char title[50];
char author[50];
int is_available;
};
struct User {
int id;
char name[50];
};
struct Library {
struct Book books[MAX_BOOKS];
struct User users[MAX_USERS];
int num_books;
int num_users;
};
void add_book(struct Library *lib) {
if (lib->num_books >= MAX_BOOKS) {
printf("The library is full!\n");
return;
}
struct Book book;
printf("Enter book title: ");
scanf("%s", book.title);
printf("Enter book author: ");
scanf("%s", book.author);
book.id = lib->num_books + 1;
book.is_available = 1;
lib->books[lib->num_books++] = book;
printf("Book added successfully!\n");
}
void display_books(struct Library *lib) {
printf("%-5s %-20s %-20s %s\n", "ID", "Title", "Author", "Availability");
for (int i = 0; i < lib->num_books; i++) {
struct Book book = lib->books[i];
printf("%-5d %-20s %-20s %s\n", book.id, book.title, book.author, book.is_available ? "Available" : "Not available");
}
}
struct Book *find_book_by_id(struct Library *lib, int id) {
for (int i = 0; i < lib->num_books; i++) {
if (lib->books[i].id == id) {
return &lib->books[i];
}
}
return NULL;
}
void borrow_book(struct Library *lib) {
int id;
printf("Enter book ID: ");
scanf("%d", &id);
struct Book *book = find_book_by_id(lib, id);
if (book == NULL) {
printf("Book not found!\n");
return;
}
if (!book->is_available) {
printf("The book is not available!\n");
return;
}
book->is_available = 0;
printf("Book borrowed successfully!\n");
}
void return_book(struct Library *lib) {
int id;
printf("Enter book ID: ");
scanf("%d", &id);
struct Book *book = find_book_by_id(lib, id);
if (book == NULL) {
printf("Book not found!\n");
return;
}
if (book->is_available) {
printf("The book is already available!\n");
return;
}
book->is_available = 1;
printf("Book returned successfully!\n");
}
void add_user(struct Library *lib) {
if (lib->num_users >= MAX_USERS) {
printf("The user list is full!\n");
return;
}
struct User user;
printf("Enter user name: ");
scanf("%s", user.name);
user.id = lib->num_users + 1;
lib->users[lib->num_users++] = user;
printf("User added successfully!\n");
}
void display_users(struct Library *lib) {
printf("%-5s %s\n", "ID", "Name");
for (int i = 0; i < lib->num_users; i++) {
struct User user = lib->users[i];
printf("%-5d %s\n", user.id, user.name);
}
}
struct User *find_user_by_id(struct Library *lib, int id) {
for (int i = 0; i < lib->num_users; i++) {
if (lib->users[i].id == id) {
return &lib->users[i];
}
}
return NULL;
}
void delete_user(struct Library *lib) {
int id;
printf("Enter user ID: ");
scanf("%d", &id);
struct User *user = find_user_by_id(lib, id);
if (user == NULL) {
printf("User not found!\n");
return;
}
// 删除用户并将其后面的用户向前移动
for (int i = 0; i < lib->num_users; i++) {
if (lib->users[i].id == id) {
for (int j = i + 1; j < lib->num_users; j++) {
lib->users[j - 1] = lib->users[j];
}
lib->num_users--;
printf("User deleted successfully!\n");
return;
}
}
}
int main() {
struct Library lib = {0};
int choice;
do {
printf("\nLibrary Menu:\n");
printf("1. Add book\n");
printf("2. Display books\n");
printf("3. Borrow book\n");
printf("4. Return book\n");
printf("5. Add user\n");
printf("6. Display users\n");
printf("7. Delete user\n");
printf("8. Exit\n");
printf("Enter your choice (1-8): ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_book(&lib);
break;
case 2:
display_books(&lib);
break;
case 3:
borrow_book(&lib);
break;
case 4:
return_book(&lib);
break;
case 5:
add_user(&lib);
break;
case 6:
display_users(&lib);
break;
case 7:
delete_user(&lib);
break;
case 8:
printf("Goodbye!\n");
exit(0);
default:
printf("Invalid choice!\n");
}
} while (1);
return 0;
}
```
希望这个修改后的代码能满足您的需求!
相关推荐
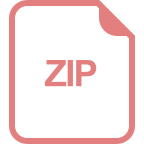
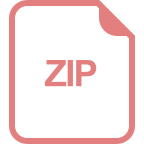
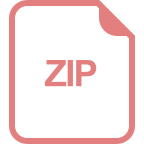














