用if-else语句和switch语句计算税金
时间: 2023-04-08 21:05:24 浏览: 166
可以回答这个问题。使用if-else语句和switch语句都可以计算税金。if-else语句可以根据不同的条件执行不同的代码块,而switch语句可以根据不同的值执行不同的代码块。具体实现方式可以根据具体需求来选择。
相关问题
写一个用if- else语句和switch语句计算税金的c++程序
以下是用if-else语句和switch语句计算税金的C程序:
使用if-else语句:
#include <stdio.h>
int main() {
float income, tax;
printf("请输入您的收入:");
scanf("%f", &income);
if (income <= 0) {
printf("您输入的收入不合法!\n");
} else if (income <= 5000) {
tax = 0;
} else if (income <= 10000) {
tax = (income - 5000) * 0.05;
} else if (income <= 20000) {
tax = 250 + (income - 10000) * 0.1;
} else if (income <= 40000) {
tax = 1250 + (income - 20000) * 0.15;
} else if (income <= 60000) {
tax = 3750 + (income - 40000) * 0.2;
} else if (income <= 80000) {
tax = 7750 + (income - 60000) * 0.25;
} else {
tax = 14750 + (income - 80000) * 0.3;
}
printf("您应缴纳的税金为:%.2f元\n", tax);
return 0;
}
使用switch语句:
#include <stdio.h>
int main() {
float income, tax;
printf("请输入您的收入:");
scanf("%f", &income);
if (income <= 0) {
printf("您输入的收入不合法!\n");
} else {
switch ((int)income / 10000) {
case 0:
tax = 0;
break;
case 1:
tax = (income - 5000) * 0.05;
break;
case 2:
case 3:
tax = 250 + (income - 10000) * 0.1;
break;
case 4:
case 5:
tax = 1250 + (income - 20000) * 0.15;
break;
case 6:
case 7:
tax = 3750 + (income - 40000) * 0.2;
break;
default:
tax = 7750 + (income - 60000) * 0.25;
break;
}
printf("您应缴纳的税金为:%.2f元\n", tax);
}
return 0;
}
if-else语句和if-else if语句详细讲解
if-else语句和if-else if语句是编程中常用的控制流语句,用于根据条件执行不同的代码块。
if-else语句的基本结构如下:
```
if (条件) {
// 条件成立时执行的代码块
} else {
// 条件不成立时执行的代码块
}
```
当条件成立时,会执行if后面的代码块;当条件不成立时,会执行else后面的代码块。注意,else部分可以省略。
if-else if语句的结构如下:
```
if (条件1) {
// 条件1成立时执行的代码块
} else if (条件2) {
// 条件2成立时执行的代码块
} else {
// 所有条件都不成立时执行的代码块
}
```
当条件1成立时,会执行if后面的代码块;当条件1不成立但条件2成立时,会执行else if后面的代码块;当所有条件都不成立时,会执行else后面的代码块。同样地,else部分可以省略。
在使用if-else语句和if-else if语句时,需要注意以下几点:
1. 条件表达式一定是布尔类型。
2. 可以嵌套使用多个if-else语句。
3. if-else if语句中,只会执行满足第一个条件的代码块。
阅读全文
相关推荐
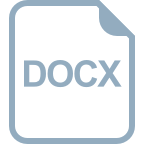
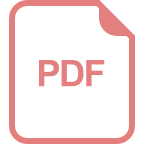
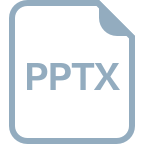













