c语言代码实现二叉链表
时间: 2023-10-18 15:04:41 浏览: 46
好的,下面是 C 语言代码实现二叉链表:
```c
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node* left;
struct node* right;
};
struct node* create_node(int data) {
struct node* new_node = (struct node*) malloc(sizeof(struct node));
new_node->data = data;
new_node->left = NULL;
new_node->right = NULL;
return new_node;
}
void insert(struct node** root, int data) {
if (*root == NULL) {
*root = create_node(data);
} else {
if (data < (*root)->data) {
insert(&((*root)->left), data);
} else {
insert(&((*root)->right), data);
}
}
}
void inorder(struct node* root) {
if (root != NULL) {
inorder(root->left);
printf("%d ", root->data);
inorder(root->right);
}
}
int main() {
struct node* root = NULL;
insert(&root, 50);
insert(&root, 30);
insert(&root, 20);
insert(&root, 40);
insert(&root, 70);
insert(&root, 60);
insert(&root, 80);
printf("Inorder traversal:\n");
inorder(root);
return 0;
}
```
这是一个简单的二叉搜索树的实现,使用了二叉链表表示法。函数 create_node 用于创建新的节点,insert 用于插入节点,inorder 用于中序遍历树。在 main 函数中,我们创建了一个二叉搜索树并进行了中序遍历。
相关推荐
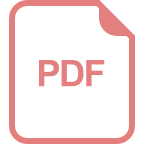
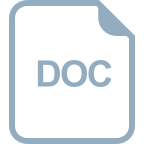














