python实现扫雷
时间: 2023-09-18 19:06:12 浏览: 115
### 回答1:
扫雷是一个经典的小游戏,以下是一个简单的Python实现:
```python
import random
# 初始化游戏棋盘
def init_board(width, height, mines):
board = [[0 for i in range(width)] for j in range(height)]
for i in range(mines):
x = random.randint(0, width - 1)
y = random.randint(0, height - 1)
while board[y][x] == -1:
x = random.randint(0, width - 1)
y = random.randint(0, height - 1)
board[y][x] = -1
for i in range(height):
for j in range(width):
if board[i][j] == -1:
continue
count = 0
if i > 0 and j > 0 and board[i - 1][j - 1] == -1:
count += 1
if i > 0 and board[i - 1][j] == -1:
count += 1
if i > 0 and j < width - 1 and board[i - 1][j + 1] == -1:
count += 1
if j > 0 and board[i][j - 1] == -1:
count += 1
if j < width - 1 and board[i][j + 1] == -1:
count += 1
if i < height - 1 and j > 0 and board[i + 1][j - 1] == -1:
count += 1
if i < height - 1 and board[i + 1][j] == -1:
count += 1
if i < height - 1 and j < width - 1 and board[i + 1][j + 1] == -1:
count += 1
board[i][j] = count
return board
# 显示游戏棋盘
def show_board(board, status):
for i in range(len(board)):
for j in range(len(board[i])):
if status[i][j] == 0:
print('_', end=' ')
elif status[i][j] == 1:
if board[i][j] == -1:
print('*', end=' ')
elif board[i][j] == 0:
print(' ', end=' ')
else:
print(board[i][j], end=' ')
else:
print('F', end=' ')
print()
# 游戏主循环
def main_loop(width, height, mines):
board = init_board(width, height, mines)
status = [[0 for i in range(width)] for j in range(height)]
show_board(board, status)
while True:
x = int(input('请输入要翻开的格子的横坐标:'))
y = int(input('请输入要翻开的格子的纵坐标:'))
if board[y][x] == -1:
print('你输了!')
status[y][x] = 1
show_board(board, status)
break
else:
status[y][x] = 1
show_board(board, status)
```
你可以通过调用 `main_loop()` 函数来开始游戏,需要输入游戏棋盘的宽度、高度和雷的数量。在游戏中,你需要输入要翻开的格子的坐标,程序会自动进行处理并显示游戏棋盘。
### 回答2:
扫雷是一个非常经典的游戏,Python可以很方便地实现扫雷游戏。
首先,我们可以创建一个二维列表作为游戏的棋盘,每个元素代表一个方块。我们可以用数字来表示每个方块的状态,比如0表示未点击过的空白方块,-1表示雷,1到8表示周围雷的数量。
接下来,我们需要实现一些函数来实现游戏的逻辑。例如,当玩家点击一个方块时,需要判断点击的方块是否是雷,如果是雷,则游戏结束,玩家失败;如果不是雷,则需要计算周围雷的数量,并显示在点击的方块上。当玩家点击一个空白方块时,需要递归地显示周围的方块,直到遇到有雷的方块为止。
另外,我们还需要实现一些辅助函数来生成雷的位置,以及判断游戏是否胜利等。
总结起来,实现扫雷游戏的关键步骤包括创建棋盘、处理玩家的点击事件、递归显示周围的方块、判断游戏是否胜利等。
Python的语法和数据结构非常适合实现扫雷游戏,而且Python还有很多库可以用来实现界面的绘制和交互。通过使用适当的数据结构和算法,我们可以很方便地实现一个简单而有趣的扫雷游戏。
阅读全文
相关推荐



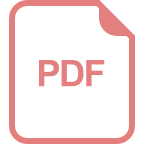
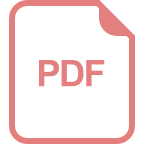
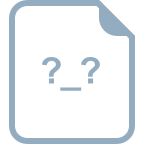



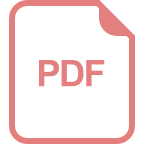
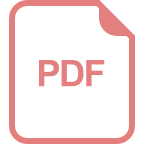
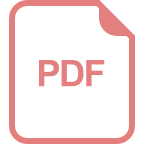