写一个从can上读取、发送数据的C++代码完整例子
时间: 2024-03-02 11:53:34 浏览: 206
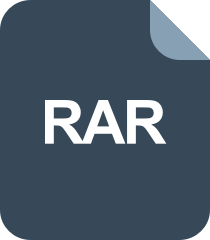
基于C#的CAN通讯示例

好的,以下是一个完整的从CAN上读取和发送数据的C++代码示例,使用了SocketCAN接口:
```c++
#include <iostream>
#include <cstring>
#include <unistd.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <net/if.h>
#include <linux/can.h>
#include <linux/can/raw.h>
using namespace std;
int main()
{
// 创建CAN套接字
int s = socket(PF_CAN, SOCK_RAW, CAN_RAW);
if (s < 0)
{
perror("socket");
return 1;
}
// 获取CAN接口的索引
struct ifreq ifr;
strcpy(ifr.ifr_name, "can0");
ioctl(s, SIOCGIFINDEX, &ifr);
// 绑定CAN套接字到CAN接口
struct sockaddr_can addr;
addr.can_family = AF_CAN;
addr.can_ifindex = ifr.ifr_ifindex;
if (bind(s, (struct sockaddr *)&addr, sizeof(addr)) < 0)
{
perror("bind");
return 1;
}
// 读取CAN数据
while (1)
{
struct can_frame frame;
int nbytes = read(s, &frame, sizeof(struct can_frame));
if (nbytes < 0)
{
perror("read");
break;
}
else if (nbytes != sizeof(struct can_frame))
{
fprintf(stderr, "read: incomplete CAN frame\n");
break;
}
cout << "Received CAN frame: id=0x" << hex << frame.can_id << ", data=";
for (int i = 0; i < frame.can_dlc; i++)
{
cout << hex << (int)frame.data[i] << " ";
}
cout << endl;
}
// 发送CAN数据
struct can_frame frame;
frame.can_id = 0x123;
frame.can_dlc = 8;
frame.data[0] = 0x11;
frame.data[1] = 0x22;
frame.data[2] = 0x33;
frame.data[3] = 0x44;
frame.data[4] = 0x55;
frame.data[5] = 0x66;
frame.data[6] = 0x77;
frame.data[7] = 0x88;
int nbytes = write(s, &frame, sizeof(struct can_frame));
if (nbytes < 0)
{
perror("write");
return 1;
}
// 关闭CAN套接字
close(s);
return 0;
}
```
上述代码中,使用了IO流库的cout输出CAN数据,方便查看。在读取CAN数据时,遍历数据数组输出每个字节的十六进制值。
该示例中发送的CAN数据帧ID为0x123,数据为8个字节的数据。发送数据时,使用write函数向CAN接口发送数据。
注意,该示例只是一个简单的示例,实际使用时需要根据具体情况进行调整和优化。
阅读全文
相关推荐
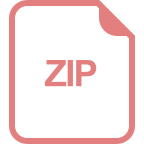
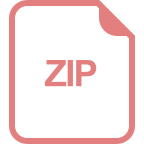
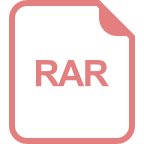
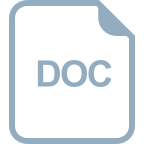
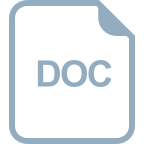
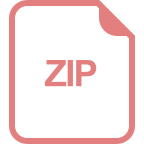
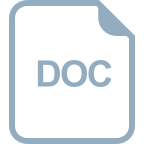
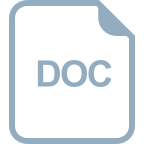
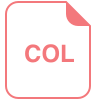






