编写Linux平台下的C应用程序,完成任务:有P1,P2,P3三个进程,P1和P2负责从键盘接收字符串,均发送给P3,P3接收到字符串,根据发送方分别显示"P3 received *** from P1(或P2)" ;分别用管道通信,消息队列和共享存储三种通信方式实现
时间: 2023-08-30 20:06:38 浏览: 82
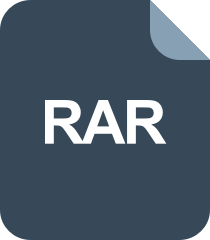
将P1口状态送入P0、P2、P3-综合文档
以下是使用管道通信方式的C应用程序实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#define MAX_BUF_SIZE 1024
int main() {
int p1[2], p2[2], nbytes;
char buf[MAX_BUF_SIZE];
if (pipe(p1) == -1 || pipe(p2) == -1) {
perror("pipe");
return 1;
}
pid_t pid = fork();
if (pid == -1) {
perror("fork");
return 1;
}
if (pid == 0) {
// P3 process
close(p1[1]);
close(p2[1]);
while (1) {
if ((nbytes = read(p1[0], buf, MAX_BUF_SIZE)) > 0) {
buf[nbytes] = '\0';
printf("P3 received %s from P1\n", buf);
}
if ((nbytes = read(p2[0], buf, MAX_BUF_SIZE)) > 0) {
buf[nbytes] = '\0';
printf("P3 received %s from P2\n", buf);
}
}
close(p1[0]);
close(p2[0]);
} else {
pid_t pid2 = fork();
if (pid2 == -1) {
perror("fork");
return 1;
}
if (pid2 == 0) {
// P2 process
close(p1[0]);
close(p2[1]);
while (1) {
printf("P2 enter a string: ");
fgets(buf, MAX_BUF_SIZE, stdin);
write(p1[1], buf, strlen(buf));
}
close(p1[1]);
close(p2[0]);
} else {
// P1 process
close(p1[1]);
close(p2[0]);
while (1) {
printf("P1 enter a string: ");
fgets(buf, MAX_BUF_SIZE, stdin);
write(p2[1], buf, strlen(buf));
}
close(p1[0]);
close(p2[1]);
}
}
return 0;
}
```
以下是使用消息队列通信方式的C应用程序实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MAX_MESSAGE_SIZE 1024
struct message {
long mtype;
char mtext[MAX_MESSAGE_SIZE];
};
int main() {
int qid;
key_t key;
struct message msg;
if ((key = ftok(".", 'a')) == -1) {
perror("ftok");
return 1;
}
if ((qid = msgget(key, IPC_CREAT | 0666)) == -1) {
perror("msgget");
return 1;
}
pid_t pid = fork();
if (pid == -1) {
perror("fork");
return 1;
}
if (pid == 0) {
// P3 process
while (1) {
if (msgrcv(qid, &msg, MAX_MESSAGE_SIZE, 1, 0) > 0) {
printf("P3 received %s from P1\n", msg.mtext);
}
if (msgrcv(qid, &msg, MAX_MESSAGE_SIZE, 2, 0) > 0) {
printf("P3 received %s from P2\n", msg.mtext);
}
}
} else {
pid_t pid2 = fork();
if (pid2 == -1) {
perror("fork");
return 1;
}
if (pid2 == 0) {
// P2 process
while (1) {
printf("P2 enter a string: ");
fgets(msg.mtext, MAX_MESSAGE_SIZE, stdin);
msg.mtype = 2;
msgsnd(qid, &msg, strlen(msg.mtext) + 1, 0);
}
} else {
// P1 process
while (1) {
printf("P1 enter a string: ");
fgets(msg.mtext, MAX_MESSAGE_SIZE, stdin);
msg.mtype = 1;
msgsnd(qid, &msg, strlen(msg.mtext) + 1, 0);
}
}
}
msgctl(qid, IPC_RMID, NULL);
return 0;
}
```
以下是使用共享存储通信方式的C应用程序实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#define MAX_BUF_SIZE 1024
int main() {
int shmid1, shmid2;
char *shm1, *shm2;
if ((shmid1 = shmget(IPC_PRIVATE, MAX_BUF_SIZE, IPC_CREAT | 0666)) == -1 ||
(shmid2 = shmget(IPC_PRIVATE, MAX_BUF_SIZE, IPC_CREAT | 0666)) == -1) {
perror("shmget");
return 1;
}
if ((shm1 = shmat(shmid1, NULL, 0)) == (char *) -1 ||
(shm2 = shmat(shmid2, NULL, 0)) == (char *) -1) {
perror("shmat");
return 1;
}
pid_t pid = fork();
if (pid == -1) {
perror("fork");
return 1;
}
if (pid == 0) {
// P3 process
while (1) {
if (*shm1 != '\0') {
printf("P3 received %s from P1\n", shm1);
*shm1 = '\0';
}
if (*shm2 != '\0') {
printf("P3 received %s from P2\n", shm2);
*shm2 = '\0';
}
}
} else {
pid_t pid2 = fork();
if (pid2 == -1) {
perror("fork");
return 1;
}
if (pid2 == 0) {
// P2 process
while (1) {
printf("P2 enter a string: ");
fgets(shm2, MAX_BUF_SIZE, stdin);
}
} else {
// P1 process
while (1) {
printf("P1 enter a string: ");
fgets(shm1, MAX_BUF_SIZE, stdin);
}
}
}
shmdt(shm1);
shmdt(shm2);
shmctl(shmid1, IPC_RMID, NULL);
shmctl(shmid2, IPC_RMID, NULL);
return 0;
}
```
阅读全文
相关推荐
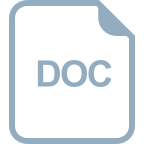
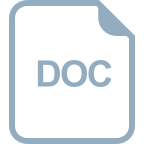
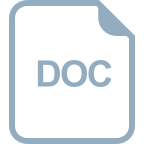













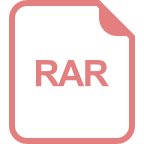