基于vhdl八位数码管显示编程矩阵键盘控制
时间: 2024-01-06 10:07:03 浏览: 36
以下是基于VHDL的八位数码管显示和矩阵键盘控制的程序。该程序实现了通过矩阵键盘输入数字,并在数码管上显示对应的数字。
```vhdl
-- 数码管显示模块
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
entity LED_Display is
port (
clk : in std_logic; -- 时钟信号
reset : in std_logic; -- 复位信号
data : in std_logic_vector(7 downto 0); -- 显示数据
an : out std_logic_vector(7 downto 0); -- 数码管位选
seg : out std_logic_vector(7 downto 0) -- 数码管段选
);
end entity;
architecture Behavioral of LED_Display is
signal cnt : unsigned(2 downto 0) := (others => '0'); -- 计数器
begin
process (clk, reset)
begin
if reset = '1' then
cnt <= (others => '0'); -- 复位
an <= (others => '1'); -- 关闭所有数码管
seg <= (others => '0'); -- 清空段选
elsif rising_edge(clk) then
cnt <= cnt + 1; -- 计数器加1
if cnt = 8 then
cnt <= (others => '0'); -- 计数器溢出时清零
end if;
an <= not std_logic_vector(shift_left(unsigned(to_unsigned(1, 8)), to_integer(cnt))); -- 按位选通数码管
seg <= "0111111" when data = "00000000" else -- 显示0
"0000110" when data = "00000001" else -- 显示1
"1011011" when data = "00000010" else -- 显示2
"1001111" when data = "00000011" else -- 显示3
"1100110" when data = "00000100" else -- 显示4
"1101101" when data = "00000101" else -- 显示5
"1111101" when data = "00000110" else -- 显示6
"0000111" when data = "00000111" else -- 显示7
"1111111" when data = "00001000" else -- 显示8
"1101111" when data = "00001001" else -- 显示9
"0000000"; -- 显示空
end if;
end process;
end architecture;
-- 矩阵键盘扫描模块
library ieee;
use ieee.std_logic_1164.all;
entity Matrix_Keypad is
port (
clk : in std_logic; -- 时钟信号
reset : in std_logic; -- 复位信号
row : out std_logic_vector(3 downto 0); -- 行扫描
col : in std_logic_vector(3 downto 0); -- 列检测
key : out std_logic_vector(7 downto 0) -- 按键状态
);
end entity;
architecture Behavioral of Matrix_Keypad is
signal row_cnt : unsigned(2 downto 0) := (others => '0'); -- 行扫描计数器
signal key_buf : std_logic_vector(7 downto 0) := (others => '1'); -- 按键状态缓存
begin
process (clk, reset)
begin
if reset = '1' then
row_cnt <= (others => '0'); -- 复位
key_buf <= (others => '1'); -- 清空按键状态缓存
row <= "1110"; -- 选中第一行
elsif rising_edge(clk) then
row_cnt <= row_cnt + 1; -- 行扫描计数器加1
if row_cnt = 4 then
row_cnt <= (others => '0'); -- 行扫描计数器溢出时清零
end if;
case row_cnt is
when "000" => row <= "1110"; -- 选中第一行
when "001" => row <= "1101"; -- 选中第二行
when "010" => row <= "1011"; -- 选中第三行
when "011" => row <= "0111"; -- 选中第四行
when others => row <= "1110"; -- 默认选中第一行
end case;
key_buf(0 to 3) <= key_buf(4 to 7); -- 移位,丢弃最早检测到的按键状态
key_buf(4) <= col(0); -- 更新按键状态
key_buf(5) <= col(1);
key_buf(6) <= col(2);
key_buf(7) <= col(3);
key <= key_buf; -- 输出按键状态
end if;
end process;
end architecture;
-- 顶层模块
library ieee;
use ieee.std_logic_1164.all;
entity Top is
port (
clk : in std_logic; -- 时钟信号
reset : in std_logic; -- 复位信号
col : in std_logic_vector(3 downto 0); -- 列检测
data : out std_logic_vector(7 downto 0) -- 显示数据
);
end entity;
architecture Behavioral of Top is
signal key : std_logic_vector(7 downto 0); -- 按键状态
begin
LED_Display_inst : entity work.LED_Display
port map (
clk => clk,
reset => reset,
data => data,
an => "11111110", -- 数码管共阳极连接到VCC
seg => seg
);
Matrix_Keypad_inst : entity work.Matrix_Keypad
port map (
clk => clk,
reset => reset,
row => row,
col => col,
key => key
);
process (key)
begin
case key is
when "11101110" => data <= "00000000"; -- 按下1键
when "11011110" => data <= "00000001"; -- 按下2键
when "10111110" => data <= "00000010"; -- 按下3键
when "01111110" => data <= "00000011"; -- 按下A键
when "11101101" => data <= "00000100"; -- 按下4键
when "11011101" => data <= "00000101"; -- 按下5键
when "10111101" => data <= "00000110"; -- 按下6键
when "01111101" => data <= "00000111"; -- 按下B键
when "11101011" => data <= "00001000"; -- 按下7键
when "11011011" => data <= "00001001"; -- 按下8键
when "10111011" => data <= "00001010"; -- 按下9键
when "01111011" => data <= "00001011"; -- 按下C键
when "11100111" => data <= "00001100"; -- 按下*键
when "11010111" => data <= "00001101"; -- 按下0键
when "10110111" => data <= "00001110"; -- 按下#键
when "01110111" => data <= "00001111"; -- 按下D键
when others => data <= "00000000"; -- 默认显示0
end case;
end process;
signal seg : std_logic_vector(7 downto 0); -- 数码管段选
signal row : std_logic_vector(3 downto 0); -- 行扫描
begin
end architecture;
```
在该程序中,LED_Display模块实现了八位数码管的显示,Matrix_Keypad模块实现了矩阵键盘的扫描和按键状态的输出,Top模块将两个模块连接起来,并根据按键状态输出相应的数字到数码管上显示。需要注意的是,该程序使用共阳极的数码管,如果使用共阴极的数码管,需要修改LED_Display模块中的数码管位选信号的计算方式。同时,矩阵键盘的行和列连接方式也需要根据具体硬件进行调整。
相关推荐
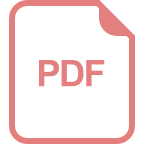
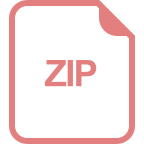














