用html写一个围棋小游戏
时间: 2023-07-20 15:39:37 浏览: 196
好的,以下是一个简单的围棋小游戏的 HTML 代码:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>围棋小游戏</title>
<style>
#board {
width: 400px;
height: 400px;
margin: 0 auto;
border: 2px solid black;
background-color: #F1C172;
position: relative;
}
.stone {
width: 20px;
height: 20px;
border-radius: 50%;
position: absolute;
cursor: pointer;
}
.black {
background-color: black;
}
.white {
background-color: white;
}
</style>
</head>
<body>
<div id="board">
<!-- 画出棋盘 -->
<div style="position: absolute; top: 0; left: 0; width: 100%; height: 100%;"></div>
<svg width="100%" height="100%">
<defs>
<pattern id="grid" width="20" height="20" patternUnits="userSpaceOnUse">
<path d="M 20 0 L 0 0 0 20" fill="none" stroke="gray" stroke-width="0.5" />
</pattern>
</defs>
<rect x="0" y="0" width="100%" height="100%" fill="url(#grid)" />
<rect x="0" y="0" width="100%" height="100%" fill="none" stroke="black" stroke-width="2" />
<g id="points"></g>
</svg>
</div>
<script>
// 获取棋盘和棋子元素
const board = document.getElementById('board');
const stones = [];
// 绘制棋盘上的点
const points = document.getElementById('points');
for (let i = 0; i < 19; i++) {
for (let j = 0; j < 19; j++) {
const point = document.createElement('circle');
point.setAttribute('cx', (i + 1) * 20);
point.setAttribute('cy', (j + 1) * 20);
point.setAttribute('r', 2);
points.appendChild(point);
}
}
// 给棋盘绑定点击事件
board.addEventListener('click', function (e) {
// 获取点击位置相对于棋盘的坐标
const x = e.offsetX;
const y = e.offsetY;
// 计算该位置对应的棋盘坐标
const i = Math.round(x / 20) - 1;
const j = Math.round(y / 20) - 1;
// 判断该位置是否已经有棋子
if (!stones[i] || !stones[i][j]) {
// 创建一个棋子元素,并添加到棋盘上
const stone = document.createElement('div');
stone.className = 'stone';
stone.style.left = i * 20 + 'px';
stone.style.top = j * 20 + 'px';
// 判断当前应该下黑子还是白子
if (stones.length % 2 === 0) {
stone.classList.add('black');
} else {
stone.classList.add('white');
}
board.appendChild(stone);
// 记录该位置已经有棋子
if (!stones[i]) {
stones[i] = [];
}
stones[i][j] = true;
}
});
</script>
</body>
</html>
```
这个小游戏使用 HTML、CSS 和 JavaScript 实现了一个简单的围棋棋盘,支持黑白两色棋子的落子。用户点击棋盘上的空位时,程序会自动判断该位置是否已经有棋子,如果没有则创建一个棋子元素,并添加到棋盘上。同时,程序会记录棋盘上所有已经有棋子的位置,以便后续判断胜负。
相关推荐
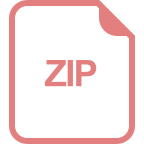
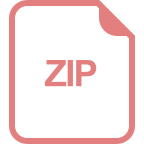














