生成python地图着色问题代码
时间: 2023-10-12 17:18:48 浏览: 124
Sure, I can help you with that. Here's an example code for generating a colored map in Python using the Matplotlib library:
```python
import numpy as np
import matplotlib.pyplot as plt
# Define the data
values = np.random.rand(10, 10)
# Define the colormap
cmap = plt.get_cmap('Greens')
# Plot the data with the colormap
plt.imshow(values, cmap=cmap)
# Show the plot
plt.show()
```
This code creates a random 10x10 array of values and uses the 'Greens' colormap to plot it as a colored map. You can modify the size of the array and use a different colormap to fit your specific needs. Let me know if you need further assistance.
相关问题
python生成栅格地图代码
当然可以!下面是一个简单的Python代码示例,于生成栅格地图:
```python
class GridMap:
def __init__(self, width, height):
self.width = width
self.height = height
self.grid = [['.' for _ in range(width)] for _ in range(height)]
def set_obstacle(self, x, y):
self.grid[y][x] = '#'
def set_start(self, x, y):
self.grid[y][x] = 'S'
def set_goal(self, x, y):
self.grid[y][x] = 'G'
def print_map(self):
for row in self.grid:
print(' '.join(row))
# 创建一个 5x5 的地图
map = GridMap(5, 5)
# 设置障碍物、起点和终点
map.set_obstacle(1, 1)
map.set_obstacle(2, 2)
map.set_start(0, 0)
map.set_goal(4, 4)
# 打印地图
map.print_map()
```
这段代码定义了一个`GridMap`类,通过调用类的方法来设置障碍物、起点和终点,并可以打印出生成的地图。你可以根据需要修改地图的大小和设置障碍物、起点和终点的位置。
地图着色问题python
地图着色问题是一个经典的图论问题,其目标是用最少的颜色对地图上的每个区域进行着色,使得相邻的区域颜色不同。在Python中,可以使用图论库NetworkX来解决这个问题。
下面是一个简单的示例代码,用于解决一个地图着色问题:
```python
import networkx as nx
# 定义一个无向图
G = nx.Graph()
# 添加节点
G.add_nodes_from(['A', 'B', 'C', 'D', 'E', 'F'])
# 添加边
G.add_edges_from([('A', 'B'), ('A', 'C'), ('B', 'C'), ('B', 'D'), ('B', 'E'), ('C', 'F'), ('D', 'E'), ('E', 'F')])
# 使用贪心算法进行着色
color_map = nx.greedy_color(G, strategy='largest_first')
# 输出着色结果
print(color_map)
```
这段代码中,我们使用了NetworkX库来定义一个无向图,然后添加节点和边。接着,我们使用贪心算法进行着色,并将结果输出。在这个例子中,我们可以看到,最少需要3种颜色才能着色每个区域,并且相邻的区域颜色不同。
阅读全文
相关推荐
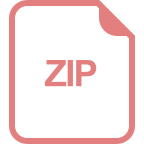
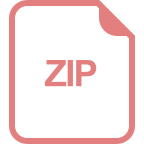
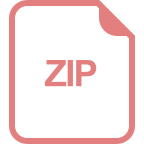













