使用Spring Security分别运用JDBC身份认证和UserDetailsService身份认证两种方式实现自定义用户认证。 提示:创建SecurityConfig类 返回AuthenticationManager
时间: 2024-02-18 11:58:59 浏览: 32
好的,您的问题是如何使用Spring Security分别运用JDBC身份认证和UserDetailsService身份认证两种方式实现自定义用户认证,并返回AuthenticationManager。
首先,我们需要创建一个SecurityConfig类,该类需要继承WebSecurityConfigurerAdapter类,并且需要使用@EnableWebSecurity注释来启用Spring Security。
接下来,我们需要实现两种不同的身份验证方式:JDBC身份认证和UserDetailsService身份认证。下面是两种实现方式的示例代码:
使用JDBC身份认证:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private DataSource dataSource;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.jdbcAuthentication()
.dataSource(dataSource)
.usersByUsernameQuery("select username, password, enabled from users where username = ?")
.authoritiesByUsernameQuery("select username, authority from authorities where username = ?");
}
}
```
使用UserDetailsService身份认证:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService);
}
}
```
在这两个示例中,我们都重写了configure(AuthenticationManagerBuilder auth)方法,并在其中实现了不同的身份验证方式。对于JDBC身份认证,我们使用了JdbcUserDetailsManager,并提供了查询用户和权限的SQL查询。对于UserDetailsService身份认证,我们使用了一个实现了UserDetailsService接口的自定义类,并将其注入到了SecurityConfig中。
最后,我们需要返回AuthenticationManager实例,以便Spring Security可以使用它来验证用户。可以通过重写configure(HttpSecurity http)方法来实现,如下所示:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private DataSource dataSource;
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.jdbcAuthentication()
.dataSource(dataSource)
.usersByUsernameQuery("select username, password, enabled from users where username = ?")
.authoritiesByUsernameQuery("select username, authority from authorities where username = ?");
auth.userDetailsService(userDetailsService);
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.logout().logoutSuccessUrl("/login?logout");
}
@Bean
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
}
```
在上面的示例中,我们使用了一个简单的HTTP安全配置,其中指定了需要登录的URL和需要拥有的角色。最后,我们重写了authenticationManagerBean()方法,并使用@Bean注释将其声明为Spring Bean,以便其他组件可以使用它来验证用户。
希望这可以回答您的问题。
相关推荐
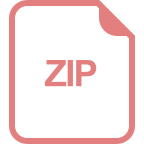
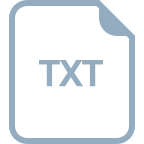
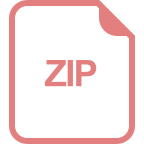
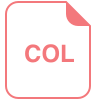
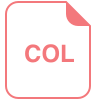
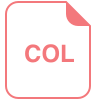
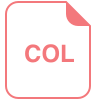
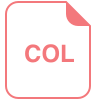









