对结构体中元素从小到大进行排序
时间: 2023-05-21 13:01:54 浏览: 138
可以使用 STL 中的 sort 函数来对结构体中的元素从小到大进行排序。具体实现方法如下:
1. 定义结构体:
```
struct Student {
string name;
int age;
double score;
};
```
2. 定义比较函数:
```
bool cmp(const Student& a, const Student& b) {
return a.score < b.score;
}
```
3. 使用 sort 函数进行排序:
```
vector<Student> students = {{"Tom", 18, 90.5}, {"Jerry", 19, 85.5}, {"Alice", 20, 95.0}};
sort(students.begin(), students.end(), cmp);
```
这样就可以将结构体中的元素按照 score 从小到大进行排序了。
相关问题
对结构体中元素进行排序
可以使用 STL 中的 sort 函数对结构体中的元素进行排序。例如,假设有一个结构体 Person 包含姓名和年龄两个元素,可以按照年龄从小到大排序,代码如下:
```
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
struct Person {
string name;
int age;
};
bool cmp(Person a, Person b) {
return a.age < b.age;
}
int main() {
vector<Person> people = {{"Tom", 20}, {"Jerry", 18}, {"Alice", 22}};
sort(people.begin(), people.end(), cmp);
for (auto p : people) {
cout << p.name << " " << p.age << endl;
}
return 0;
}
```
输出结果为:
```
Jerry 18
Tom 20
Alice 22
```
其中,cmp 函数为自定义的比较函数,用于指定排序规则。在本例中,按照年龄从小到大排序。
C语言利用直接插入排序算法,将顺序表L中的元素从小到大进行排序
好的,下面是C语言利用直接插入排序算法,将顺序表L中的元素从小到大进行排序的代码:
```c
void InsertSort(SqList *L)
{
int i, j;
for (i = 2; i <= L->length; i++) // 从第二个元素开始插入
{
if (L->r[i] < L->r[i - 1]) // 如果当前元素小于前一个元素
{
L->r[0] = L->r[i]; // 设置哨兵
for (j = i - 1; L->r[j] > L->r[0]; j--) // 从后往前查找插入位置
{
L->r[j + 1] = L->r[j]; // 后移元素
}
L->r[j + 1] = L->r[0]; // 插入当前元素
}
}
}
```
其中,SqList为顺序表的结构体:
```c
#define MAXSIZE 100 // 定义顺序表的最大长度
typedef struct {
int r[MAXSIZE + 1]; // 用于存储顺序表中的元素,r[0]用作哨兵或临时变量
int length; // 顺序表的长度
} SqList;
```
需要注意的是,这里使用了哨兵法,将当前元素保存在哨兵L->r[0]中,可以避免每次查找插入位置时都需要进行边界判断。
相关推荐
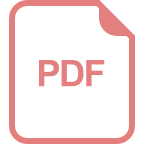
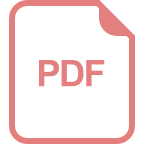
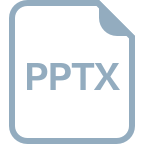












