c++一个结构体数组包含x和 y两个元素,根据x的大小从小到大排序,
时间: 2024-09-16 19:01:03 浏览: 41
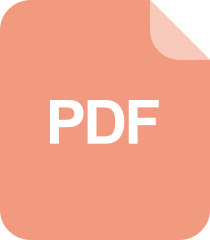
C#调用C++DLL传递结构体数组的终极解决方案
在C++中,你可以使用标准库中的`std::sort`函数来对结构体数组按`x`字段进行升序排序。假设你的结构体名为`MyStruct`,它有两个成员变量`x`和`y`,可以按照以下步骤操作:
```cpp
#include <algorithm> // 包含sort函数
// 假设MyStruct的定义如下
struct MyStruct {
int x;
int y;
};
// 定义一个MyStruct数组
MyStruct arr[] = { ... }; // 初始化数组内容
size_t array_size = sizeof(arr) / sizeof(arr[0]);
// 自定义比较函数,用于告诉sort函数如何比较x值
bool compare(const MyStruct &a, const MyStruct &b) {
return a.x < b.x; // 按照x的值升序排列
}
// 使用sort函数并传递自定义比较函数
std::sort(arr, arr + array_size, compare);
```
完成上述代码后,数组`arr`就会按照`x`字段的值从小到大排序了。
阅读全文
相关推荐
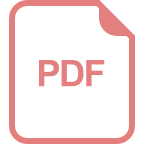
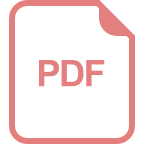











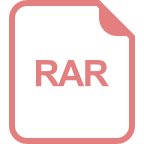
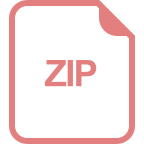
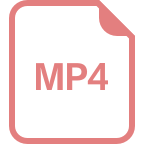