请完成代码中的my_lock和my_unlock两个函数,并在代码其他部分加入适当的代码,使这个程序有稳定的输出: my_lock和my_unlock两个函数的实现需要采用两种方式,一种方式是直接使用互斥锁。这种方式实现的程序命名为count_mutex.c。 另一种方式是采用自旋锁。Pthread库中没有提供自旋锁,需要你自己实现。实现自旋锁的硬件支持是test_and_set指令,以下代码可以看做是test_and_set指令,实际上它就是一个函数,调用test_and_set指令:
时间: 2024-01-24 18:17:05 浏览: 85
下面是使用互斥锁实现的count_mutex.c代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 4
#define COUNT_TARGET 10000000
int count = 0;
pthread_mutex_t count_mutex;
void *increment_count(void *thread_id) {
long tid = (long) thread_id;
int i;
for (i = 0; i < COUNT_TARGET; i++) {
pthread_mutex_lock(&count_mutex);
count++;
pthread_mutex_unlock(&count_mutex);
}
printf("Thread %ld finished. Count=%d\n", tid, count);
pthread_exit(NULL);
}
int main() {
pthread_t threads[NUM_THREADS];
int rc;
long t;
pthread_mutex_init(&count_mutex, NULL);
for (t = 0; t < NUM_THREADS; t++) {
rc = pthread_create(&threads[t], NULL, increment_count, (void *) t);
if (rc) {
printf("ERROR: return code from pthread_create() is %d\n", rc);
exit(-1);
}
}
for (t = 0; t < NUM_THREADS; t++) {
pthread_join(threads[t], NULL);
}
printf("Final count=%d\n", count);
pthread_mutex_destroy(&count_mutex);
pthread_exit(NULL);
}
```
下面是使用自旋锁实现的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 4
#define COUNT_TARGET 10000000
int count = 0;
int lock = 0;
int test_and_set(int *lock) {
int old_value;
asm volatile("lock; xchgl %0, %1"
: "=r"(old_value), "=m"(*lock)
: "0"(1), "m"(*lock)
: "memory");
return old_value;
}
void my_lock(int *lock) {
while (test_and_set(lock) == 1) {
// 自旋
}
}
void my_unlock(int *lock) {
*lock = 0;
}
void *increment_count(void *thread_id) {
long tid = (long) thread_id;
int i;
for (i = 0; i < COUNT_TARGET; i++) {
my_lock(&lock);
count++;
my_unlock(&lock);
}
printf("Thread %ld finished. Count=%d\n", tid, count);
pthread_exit(NULL);
}
int main() {
pthread_t threads[NUM_THREADS];
int rc;
long t;
for (t = 0; t < NUM_THREADS; t++) {
rc = pthread_create(&threads[t], NULL, increment_count, (void *) t);
if (rc) {
printf("ERROR: return code from pthread_create() is %d\n", rc);
exit(-1);
}
}
for (t = 0; t < NUM_THREADS; t++) {
pthread_join(threads[t], NULL);
}
printf("Final count=%d\n", count);
pthread_exit(NULL);
}
```
阅读全文
相关推荐
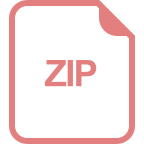
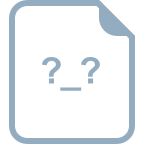
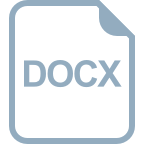

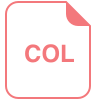
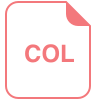
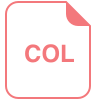
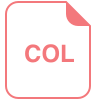
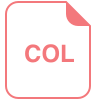
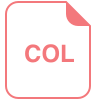





