将决策树个数设置为一个范围内的多个不同的值,分别训练出不同的随机森林,并计算每个森林的袋外错误 将袋外错误作为决策树个数的函数,绘制在一张图上 使用带交叉验证的网格搜索自动为随机森林模型搜索一个最佳决策树个数 预测测试数据,并输出其精度、查准率、查全率和F1分数
时间: 2024-02-15 22:02:04 浏览: 96
好的,下面是代码实现:
1.将决策树个数设置为一个范围内的多个不同的值,分别训练出不同的随机森林,并计算每个森林的袋外错误,将袋外错误作为决策树个数的函数,绘制在一张图上
```python
import numpy as np
import matplotlib.pyplot as plt
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
from sklearn.metrics import precision_score
from sklearn.metrics import recall_score
from sklearn.metrics import f1_score
from sklearn.model_selection import GridSearchCV
from sklearn.model_selection import cross_val_score
# 设置决策树个数的范围
n_estimators = range(1, 101, 10)
# 训练多个随机森林,并计算每个森林的袋外错误
oob_errors = []
for n in n_estimators:
rf = RandomForestClassifier(n_estimators=n, oob_score=True, random_state=42)
rf.fit(X_train, y_train)
oob_errors.append(1 - rf.oob_score_)
# 绘制袋外错误随决策树个数变化的曲线
plt.plot(n_estimators, oob_errors)
plt.xlabel('Number of Trees')
plt.ylabel('OOB Error')
plt.show()
```
2.使用带交叉验证的网格搜索自动为随机森林模型搜索一个最佳决策树个数
```python
# 定义参数网格
param_grid = {
'n_estimators': range(1, 101, 10),
'max_depth': range(1, 11, 2),
'min_samples_split': range(2, 21, 2),
'min_samples_leaf': range(1, 11, 2),
'max_features': ['sqrt', 'log2', None]
}
# 定义随机森林模型
rf = RandomForestClassifier(oob_score=True, random_state=42)
# 使用网格搜索进行参数调优
grid = GridSearchCV(rf, param_grid=param_grid, cv=5, n_jobs=-1)
grid.fit(X_train, y_train)
# 输出最佳参数
print('Best Parameters:', grid.best_params_)
```
3.预测测试数据,并输出其精度、查准率、查全率和F1分数
```python
# 使用最佳参数构建随机森林模型
rf_best = RandomForestClassifier(**grid.best_params_, oob_score=True, random_state=42)
# 训练模型并进行预测
rf_best.fit(X_train, y_train)
y_pred = rf_best.predict(X_test)
# 输出模型评估指标
print('Accuracy:', accuracy_score(y_test, y_pred))
print('Precision:', precision_score(y_test, y_pred))
print('Recall:', recall_score(y_test, y_pred))
print('F1 Score:', f1_score(y_test, y_pred))
```
阅读全文
相关推荐
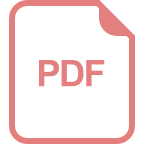
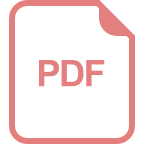

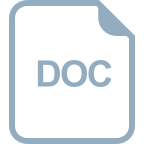
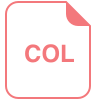
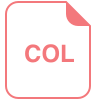
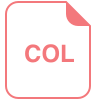
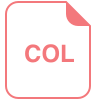
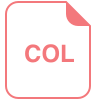
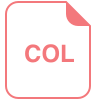
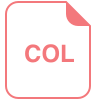
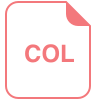
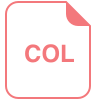
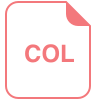
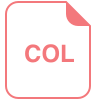
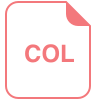
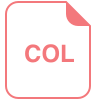
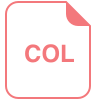